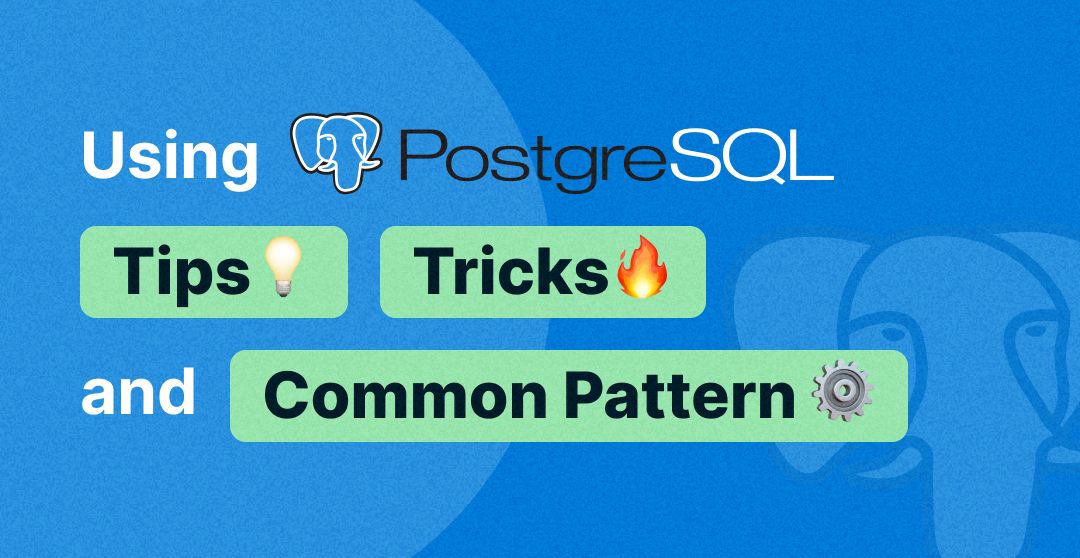
Using PL/pgSQL: Tips, Tricks, and Common Patterns
PL/pgSQL is PostgreSQL’s native procedural language. It allows developers to create custom functions, procedures, triggers, and more to handle complex tasks within the database. Mastering PL/pgSQL can lead to more robust, efficient, and maintainable database solutions. This article aims to provide an in-depth guide to PL/pgSQL, covering its syntax, best practices, and optimization techniques.
Setting the Stage: Basic Concepts
What is PL/pgSQL?
PL/pgSQL stands for “Procedural Language/PostgreSQL.” Unlike SQL, which is a declarative language focused on defining what you want to do with data, PL/pgSQL is a procedural language, meaning it defines how you want to manipulate the data. You can create custom functions, triggers, and stored procedures, giving you far greater control over your database interactions.
PL/pgSQL is especially useful in scenarios requiring complex calculations, data transformations, or procedural logic that would be cumbersome or inefficient to handle with plain SQL. For example, in financial applications, PL/pgSQL can be used to perform complex interest rate calculations. In e-commerce platforms, it can be used to manage inventory levels and pricing algorithms.
Advantages of PL/pgSQL
- Reusable code blocks can be encapsulated into functions and procedures, making it easier to manage and maintain code.
- PL/pgSQL supports transactional operations, allowing for rollbacks in case of errors, thus ensuring data integrity.
- Implement business rules and complex logic directly in the database layer, reducing application complexity and the need for multiple round-trips between the database and application layers.
PL/pgSQL supports a rich set of data types including integers, decimals, strings, and booleans, among others. This facilitates complex data manipulations and logical operations. Moreover, it allows a wide variety of control structures like loops, conditional statements, and exception handling, making it a versatile tool for any developer.
PL/pgSQL can enforce access controls and data validations, thus providing an additional layer of security. This is particularly important for ensuring that only authorized users can access or modify specific portions of the data.
The Basics: PL/pgSQL Syntax and Constructs
Understanding the fundamental syntax and constructs of PL/pgSQL is akin to mastering the building blocks of a language. By doing so, you can create efficient, robust, and maintainable database procedures. In this section, we will dive deep into these building blocks.
Variables and Data Types
PL/pgSQL supports a variety of native PostgreSQL data types, as well as user-defined types. Variables in PL/pgSQL are strongly typed, and they need to be declared in the DECLARE section of a PL/pgSQL block.
- Basic Types: INTEGER, TEXT, BOOLEAN, etc.
- Composite Types: RECORD, %ROWTYPE
- Special Variables: FOUND, NOTFOUND
Here’s how you can declare variables:
DECLARE age INTEGER := 25; name TEXT := 'John'; is_active BOOLEAN := TRUE; BEGIN -- your code END;
Control Structures (IF, CASE, LOOP)
Control structures are crucial for incorporating logic and flow into your PL/pgSQL programs.
- Conditional Statements: IF, ELSIF, and ELSE allow for branching logic.
IF age > 21 THEN -- permissible activities ELSIF age > 17 THEN -- limited activities ELSE -- not permissible END IF;
- Looping Constructs: FOR, WHILE, and LOOP enable iterative operations.
FOR i IN 1..10 LOOP -- repetitive operation END LOOP;
- CASE Statements: These are useful for handling multiple conditions more readably than using nested IF-THEN-ELSE statements.
CASE age WHEN 21 THEN -- operation WHEN 18 THEN -- operation ELSE -- default operation END CASE;
Error Handling and Exceptions
PL/pgSQL provides a robust mechanism to catch and handle errors. The BEGIN … EXCEPTION … END block allows for sophisticated error trapping and handling.
- Predefined Exceptions: Like unique_violation, foreign_key_violation, etc.
- User-defined Exceptions: Raise custom exceptions for application-specific issues.
BEGIN -- risky operation EXCEPTION WHEN unique_violation THEN -- handle error END;
Cursors and Record Processing
PL/pgSQL supports cursors for traversing record sets, typically within a loop. Cursors are generally used when you’re dealing with multiple rows and performing operations that cannot be achieved through a single SQL query.
- Explicit Cursors: Declared and controlled manually.
- Implicit Cursors: Automatically managed by PL/pgSQL in FOR loops.
DECLARE my_cursor CURSOR FOR SELECT * FROM students; student_record students%ROWTYPE; BEGIN OPEN my_cursor; LOOP FETCH my_cursor INTO student_record; EXIT WHEN NOT FOUND; -- process each record END LOOP; CLOSE my_cursor; END;
By gaining proficiency in these essential PL/pgSQL constructs, you establish a strong foundation for writing advanced procedures, functions, and triggers. Mastering these basics enables you to create more complex and efficient operations, unlocking the full potential of what you can do within the PostgreSQL database.
Tips for Effective PL/pgSQL Coding
Writing effective PL/pgSQL code not only enhances performance but also significantly eases the maintenance and scalability of your database applications. Below are some tips that can guide you to write cleaner, more efficient, and more maintainable PL/pgSQL code.
Modularize Code
Breaking down complex code into reusable functions and procedures is the essence of modular programming. This aids in reducing redundancy and makes it easier to manage and debug code.
- Function: Designed to return a value and possibly have side effects.
- Procedure: Designed for side effects and does not return a value.
For instance, if you frequently calculate a customer’s lifetime value in various parts of your application, encapsulate this logic within a function.
CREATE OR REPLACE FUNCTION calculate_lifetime_value(customer_id INTEGER) RETURNS FLOAT AS $$ BEGIN -- Calculation logic here RETURN lifetime_value; END; $$ LANGUAGE plpgsql;
Documentation and Comments
Proper documentation is vital for the long-term maintainability of code. Well-placed comments explaining the why behind a piece of code can be invaluable.
- Use block comments to describe the purpose and behavior of functions and procedures.
- Use inline comments judiciously to clarify complex lines of code.
/* This function calculates and returns the lifetime value of a customer based on their purchase history. */ CREATE OR REPLACE FUNCTION …
Error Handling
Error handling in PL/pgSQL is not merely about capturing exceptions but also about taking appropriate actions such as logging, alerting, or even triggering other processes.
- Use RAISE NOTICE for debugging or informational messages.
- Use RAISE EXCEPTION to propagate errors up the stack.
BEGIN -- some operation EXCEPTION WHEN division_by_zero THEN RAISE WARNING 'Division by zero attempted.'; -- handle error gracefully END;
Use Locking Mechanisms Wisely
PL/pgSQL allows for various types of locks like row-level locks, table-level locks, and advisory locks. Proper use of these mechanisms can help avoid deadlocks and improve data consistency.
SELECT * FROM table_name FOR UPDATE; -- Row-level lock LOCK TABLE table_name IN SHARE MODE; -- Table-level lock
Leverage RETURNING Clauses
When performing data manipulation operations like INSERT, UPDATE, or DELETE, using the RETURNING clause can reduce the need for making an additional query to retrieve data.
UPDATE employees SET salary = salary * 1.10 WHERE employee_id = 1 RETURNING salary;
These tips serve as a framework that can guide you through complex database projects, enabling you to write code that stands the test of time.
Common Patterns in PL/pgSQL
While PL/pgSQL provides a broad range of features for custom database programming, certain patterns emerge as particularly useful in regular development activities. These patterns encapsulate best practices, simplify coding, and often provide more efficient ways to perform common tasks. Below are some widely-used PL/pgSQL patterns that could benefit almost any database project.
Data Validation and Sanitization
One recurring theme in database programming is the need to validate and sanitize incoming data before performing any operations on it.
- Pattern: Use PL/pgSQL functions to perform validation and cleaning tasks before inserting or updating records.
CREATE OR REPLACE FUNCTION validate_and_insert_product(name TEXT, price NUMERIC) RETURNS VOID AS $$ BEGIN IF name IS NULL OR price IS NULL THEN RAISE EXCEPTION 'Invalid input values'; END IF; -- Further validation logic INSERT INTO products (name, price) VALUES (name, price); END; $$ LANGUAGE plpgsql;
Batch Processing
When dealing with large datasets, batch processing is a common requirement.
- Pattern: Use cursors and loops to divide the job into manageable chunks, improving both memory consumption and speed.
DECLARE batch_size INTEGER := 1000; my_cursor CURSOR FOR SELECT * FROM large_table; BEGIN FOR record IN my_cursor LIMIT batch_size LOOP -- Process each record END LOOP; END;
Audit Logging
For maintaining a history of changes to critical data, audit logging is commonly used.
- Pattern: Create triggers to log changes automatically whenever insert, update, or delete operations occur on a particular table.
CREATE TRIGGER log_changes AFTER INSERT OR UPDATE OR DELETE ON important_table FOR EACH ROW EXECUTE FUNCTION log_audit();
Dynamic SQL Execution
Sometimes, you may need to construct SQL queries dynamically based on various conditions.
- Pattern: Use EXECUTE for dynamic SQL execution within PL/pgSQL.
DECLARE dynamic_query TEXT; BEGIN dynamic_query := 'SELECT * FROM ' || table_name; EXECUTE dynamic_query; END;
Hierarchical Data Handling
Database tables often represent hierarchical or tree-like data structures.
- Pattern: Use recursive Common Table Expressions (CTEs) to traverse such structures effectively.
WITH RECURSIVE org_chart AS ( SELECT id, name, manager_id FROM employees WHERE manager_id IS NULL UNION ALL SELECT e.id, e.name, e.manager_id FROM employees e INNER JOIN org_chart o ON o.id = e.manager_id ) SELECT * FROM org_chart;
Bulk Data Transformations
Transforming large sets of data is a common need, often requiring complex SQL queries.
- Pattern: Encapsulate transformation logic in PL/pgSQL functions or procedures for better performance and reusability.
CREATE OR REPLACE FUNCTION bulk_transform() RETURNS VOID AS $$ BEGIN UPDATE table1 SET column1 = ... WHERE condition; DELETE FROM table2 WHERE condition; -- More transformations END; $$ LANGUAGE plpgsql;
Understanding these common patterns provides you with a toolkit of proven techniques that can make your PL/pgSQL programming more efficient, clean, and maintainable. They serve as a starting point and can be adapted as per the specific requirements of your application.
Debugging and Profiling PL/pgSQL
Debugging Techniques
Debugging in PL/pgSQL may involve tracing errors, checking variable values, or understanding the flow of PL/pgSQL blocks.
- RAISE Statements: The RAISE statement can be used for debugging by generating custom notices, warnings, or exceptions. This helps to get insights into variable values or flow of execution.
RAISE NOTICE 'The value of variable x is %', x;
- SQL-level Debugging: Some IDEs and third-party tools offer SQL-level debugging. This allows you to step through PL/pgSQL code line-by-line, much like you would in other programming languages.
- Logging: Utilize PostgreSQL’s robust logging features to capture detailed logs that can be analyzed for debugging.
-- Enable detailed logging in PostgreSQL SET client_min_messages TO DEBUG;
Profiling Tools
Profiling helps you understand the performance characteristics of your PL/pgSQL code, such as identifying slow-running queries or resource-intensive operations.
- EXPLAIN: Though more SQL-focused, the EXPLAIN command can help you understand how a query within a PL/pgSQL function is executed, offering insights into potential bottlenecks.
EXPLAIN ANALYZE SELECT * FROM table_name WHERE column = value;
- pg_stat_statements: This extension provides a means to track execution statistics of all SQL statements, helping you identify inefficient queries within your PL/pgSQL code.
SELECT * FROM pg_stat_statements;
- Custom Profiling: For more specific performance metrics, you can use custom PL/pgSQL functions to record start and end times for various operations, and then calculate the time elapsed.
DECLARE start_time TIMESTAMP; BEGIN start_time := clock_timestamp(); -- Code to profile RAISE NOTICE 'Elapsed time: %', clock_timestamp() - start_time; END;
Transaction Analysis
Analyzing transactions can help debug issues related to data integrity, deadlocks, or other transactional behaviors.
- pg_locks: This system view can help you analyze locks within your transactions, assisting in debugging deadlocks or long-wait scenarios.
SELECT * FROM pg_locks WHERE granted IS FALSE;
- Serializable Isolation: If your PL/pgSQL code involves complex transactions, setting the appropriate transaction isolation level can help debug issues related to concurrency.
SET TRANSACTION ISOLATION LEVEL SERIALIZABLE;
Effective debugging and profiling in PL/pgSQL require a mixture of native PL/pgSQL capabilities, PostgreSQL system views, and third-party tools. Being proficient in these methods allows you to not only find and fix issues more effectively but also to write optimized and efficient code from the outset.
FAQ
Q: What are some best practices for effective PL/pgSQL coding?
Answer: Effective PL/pgSQL coding involves several best practices, such as modularizing code into reusable functions and procedures, providing comprehensive documentation and comments, and implementing robust error handling. Leveraging appropriate locking mechanisms and using the RETURNING clause for data manipulation are also recommended.
Q: Can you name some common patterns in PL/pgSQL coding?
Answer: Common PL/pgSQL patterns include data validation and sanitization, batch processing for large datasets, and audit logging for tracking changes to critical data. Other patterns involve the use of dynamic SQL execution through the EXECUTE statement, hierarchical data handling via recursive Common Table Expressions (CTEs), and bulk data transformations encapsulated within functions or procedures.
Q: What debugging techniques are available in PL/pgSQL?
Answer: PL/pgSQL offers several debugging techniques, such as the use of RAISE statements to trace errors and monitor variable values, SQL-level debugging in supported IDEs, and PostgreSQL’s native logging features. These methods provide insights into the runtime behavior of the code and help diagnose issues.
Q: How can one profile PL/pgSQL code for performance?
Answer: Profiling in PL/pgSQL can be done using the EXPLAIN command to analyze the execution plan of SQL queries, monitoring execution statistics using the pg_stat_statements extension, and custom profiling to measure the execution time of specific code blocks. Transaction analysis via system views like pg_locks can also be valuable for understanding performance bottlenecks.
Q: How can one handle hierarchical data in PL/pgSQL?
Answer: Handling hierarchical data effectively in PL/pgSQL usually involves the use of recursive Common Table Expressions (CTEs). These allow you to traverse tree-like or hierarchical data structures stored in tables, and they can be particularly useful in scenarios like organizational charts, category trees, or any nested relationships.
Conclusion
Mastering PL/pgSQL extends your PostgreSQL capabilities significantly, allowing for better optimization, maintainability, and functionality. To further your understanding, engage with the community through forums, read up on official documentation, and continue experimenting.
By understanding and applying these tips, tricks, and common patterns, you can make the most out of what PL/pgSQL has to offer in your PostgreSQL database environments.