String manipulation in Python is an essential skill for anyone looking to boost their programming prowess—especially for data engineers and career changers. Whether you’re parsing data, formatting output, or even cleaning text, getting comfortable with string manipulation can make a big difference in your coding confidence.
In this post, we’re going to tackle five practical string manipulation problems. Each challenge will help solidify your understanding and enhance your problem-solving skills. You might wonder, why is this important? Mastering these concepts can prepare you for the everyday tasks you’ll face in real-world projects.
If you’re serious about advancing your skills, consider exploring our personalized training options that cater to your unique learning path. Also, check out our YouTube channel for more resources and tips on programming. Let’s dive in and get hands-on with Python!
Understanding String Manipulation in Python
String manipulation is a fundamental skill in Python programming, particularly for data engineers. It involves altering, processing, and analyzing text strings to extract meaningful data. Mastering string manipulation is crucial for several reasons. First, it enables efficient data processing. In practical terms, this means transforming raw data into a usable format—vital in real-world applications like data analytics. Whether you’re cleaning text data or preparing it for storage and further analysis, a solid grasp of string manipulation can streamline your workflows and enhance productivity.
Definition and Importance
At its core, string manipulation refers to modifying or analyzing sequences of characters. This includes operations like concatenation, slicing, searching, and formatting. Why does understanding this matter? Well, data comes in various forms, but much of it is text-based. From user input to file content, if you can’t effectively handle strings, you limit your ability to extract insights from your data.
Consider this: when cleaning data, you often face inconsistent formats. You might need to trim whitespace, replace special characters, or extract specific patterns. These tasks may seem straightforward, but precise string manipulation turns chaotic data into organized information. Whether you’re preparing data for analysis, debugging code, or simply formatting outputs for better readability, string manipulation is inherently intertwined with your daily programming tasks.
Common Use Cases
String manipulation plays a pivotal role across many data engineering tasks. Here are some common applications:
- Data Cleaning: This often involves removing unwanted characters, standardizing formats, and correcting inconsistencies. For example, you may need to strip leading and trailing spaces from user inputs or convert all text to lowercase.
- Data Transformation: Transforming data includes formatting strings to suit different requirements, such as changing a date format or converting CSV data into JSON.
- Searching for Patterns: Using regular expressions (regex) allows you to locate specific patterns within strings. This can be incredibly useful when handling unstructured data.
- String Formatting: When generating reports or user interfaces, you need to format strings for clarity—like embedding variables into templates or creating user-friendly messages.
- Parsing Data: Extracting information from complex strings is a common task. Imagine parsing a CSV file where each line contains a distinct record, and you need to separate these values from their delimiters.
As you enhance your programming confidence, diving deeper into string manipulation challenges will only solidify your skills. Want more practical exercises? Check out our personalized training options designed to cater to your unique learning path or explore our YouTube channel for additional resources.
Problem 1: Reversing a String
Reversing a string is a common problem that tests your understanding of Python syntax and comprehension of how strings work. It may appear simple, but there are several effective methods to achieve this, each with its own advantages. Let’s explore these methods step-by-step and understand their practical applications, especially in the field of data engineering.
Step-by-Step Solution
There are multiple ways to reverse a string in Python. Here’s a detailed look at some of the most common methods:
- Using Slicing: Slicing is one of the most efficient ways to reverse a string. Here’s how it works:
original_string = "Hello, World!" reversed_string = original_string[::-1] print(reversed_string) # Output: !dlroW ,olleH
This method utilizes Python’s slicing capabilities. The[::-1]
slice notation effectively tells Python to start from the end of the string and move backward. - Using the
reversed()
Function: Python provides a built-inreversed()
function. While it does return an iterator, you can join the characters back into a string like this:original_string = "Hello, World!" reversed_string = ''.join(reversed(original_string)) print(reversed_string) # Output: !dlroW ,olleH
This method is helpful when you want to maintain a clear, readable approach. - Using a Loop: This approach is a bit more manual but very informative for understanding string manipulation:
original_string = "Hello, World!" reversed_string = '' for char in original_string: reversed_string = char + reversed_string print(reversed_string) # Output: !dlroW ,olleH
It builds the reversed string character by character, which can be great for teaching as it emphasizes how iteration works. - Using Recursion: For those who enjoy a challenge, reversing a string using recursion can be quite beautiful:
def reverse_string(s): if len(s) == 0: return s return s[-1] + reverse_string(s[:-1]) print(reverse_string("Hello, World!")) # Output: !dlroW ,olleH
This method teaches fundamental concepts of recursion, which is essential in programming.
Each method has its place, depending on the context in which you’re working.
Practical Application
Reversing strings isn’t just a coding exercise; it has practical applications, particularly in data engineering. Here are a few scenarios where he can prove useful:
- Data Parsing: When processing texts with reversed segments (like timestamps or special identifiers), reversing a string can help isolate and retrieve the necessary data segments.
- Data Validation: In certain cases, you’ll have to check if a string is a palindrome, which involves reversing the string and comparing it to the original. This can often be crucial in ensuring data consistency.
- Encoding/Decoding: If you’re working with encryption or encoding methods, you might need to reverse strings as part of the algorithmic process. Understanding how to manipulate strings effectively is foundational for these tasks.
- Text Processing: When working with data souces like logs or datasets formatted in unconventional ways, reversing strings can help reformat text into a more usable structure.
Mastering these string manipulation challenges will not only build your programming confidence but also prepare you for the everyday tasks you’ll encounter as a data engineer. Want to enhance your skills even further? Consider checking out our personalized training options or explore our YouTube channel for more insights on Python programming.
Problem 2: Finding Substrings
When it comes to working with strings in Python, finding substrings is a key skill every data engineer must master. The ability to search for and validate substrings can dramatically enhance your ability to handle data efficiently. Whether you’re cleaning datasets, logging information, or even preparing text for analysis, understanding how to locate substrings positions you for success. Let’s break down the methods available and their applications.
Using Python’s Built-in Methods
Python offers several built-in methods to help you find substrings with ease. Here are some of the most effective ways to get the job done, along with tips on how to handle common edge cases:
- The
in
Operator: This is one of the simplest methods. You can check if a substring exists within a string using this straightforward syntax:main_string = "Data Engineering in Python" substring = "Python" found = substring in main_string print(found) # Output: True
It returnsTrue
if found, which makes it concise for validation checks. - The
find()
Method: If you want to know the starting index of your substring, usefind()
. It returns-1
if not found:index = main_string.find(substring) print(index) # Output: 19
This can be particularly useful when you need to know where the substring occurs in your main string. - The
index()
Method: Similar tofind()
, butindex()
raises aValueError
if the substring is not found, which can be useful for debugging:try: index = main_string.index(substring) print(index) except ValueError: print("Substring not found")
- Regular Expressions (Regex): For more complex substring searches, Python’s
re
library provides powerful capabilities. Whether you’re looking for patterns or validating formats, regex can handle much more complicated searches:import re pattern = r"Data.*Python" match = re.search(pattern, main_string) if match: print("Match found!")
When finding substrings, edge cases might include empty strings or strings with special characters. Always consider these possibilities in your code to avoid unexpected errors or results.
Real-World Examples
Finding substrings is not just about academic exercises; it’s critical in various practical scenarios, especially in data processing:
- Data Cleaning: When handling large datasets, you might need to filter data or remove records based on specific keywords. For instance, locating rows containing a specific substring in a customer feedback file can help flag particular feedback for analysis.
- Log Analysis: In monitoring applications, logging data often includes strings where specific error codes or keywords must be found. Searching for these substrings allows engineers to pinpoint issues in overwhelming datasets.
- Natural Language Processing (NLP): When working with text data in NLP, identifying common phrases or tokenizing sentences is fundamental. This can help improve search functionalities or text classification.
- Data Validation: If you’re validating input from users, searching for substrings can reveal inconsistencies. For instance, checking if a user input contains valid commands or options ensures that your application can respond appropriately.
Mastering how to find substrings not only boosts your programming confidence but also equips you for real-world challenges in data engineering. If you’re eager to elevate your skills further, explore our personalized training options that adapt to your learning style. Want more insights? Check out our YouTube channel for engaging tutorials and discussions.
Problem 3: String Formatting
String formatting is a cornerstone of programming in Python and an important skill for data engineers. Knowing how to manipulate string outputs effectively can help you enhance the readability of your data presentations and reports. Let’s explore how you can elevate your Python skills by mastering different string formatting techniques and understanding how they can be applied in real-world scenarios.
Different Formatting Techniques
In Python, there are several ways to format strings, each with its own advantages. Here’s a rundown of the most popular techniques:
- F-Strings: Introduced in Python 3.6, f-strings allow you to embed expressions inside string literals, using curly braces. This makes them concise and easy to read:
name = "Alice" age = 30 message = f"{name} is {age} years old." print(message) # Output: Alice is 30 years old.
str.format()
Method: This method provides a flexible way to format strings by using placeholders. You can control the output format in various ways:name = "Bob" score = 87.5 message = "{} scored {:.1f} out of 100.".format(name, score) print(message) # Output: Bob scored 87.5 out of 100.
- Percent Formatting: This older method uses the percent operator for formatting. While it’s less common now, it’s still worth noting for legacy codebases:
temperature = 23.5 print("The temperature is %.1f degrees." % temperature) # Output: The temperature is 23.5 degrees.
By mastering these string formatting techniques, you can make your code cleaner and more understandable, allowing other developers (or your future self) to read it with ease.
Use Cases in Data Engineering
String formatting plays a significant role in data engineering, particularly when generating outputs for reports and dashboards. Let’s explore some practical situations where effective string formatting is crucial:
- Generating Reports: When creating summary reports from large datasets, formatted strings can enhance clarity. For instance, when reporting average sales data, a well-formatted table using f-strings or the
format()
method will greatly improve readability. - Logging Information: Good logs are essential for debugging. Using formatted strings for log messages allows you to include variables and dynamic content. This is crucial when running data pipelines:
import logging user = "admin" action = "update" logging.info(f"{user} performed an {action} action.")
- Preparing Outputs for APIs: When working with APIs, structured output is essential. You may need to format strings as JSON or XML. Understanding how to format your strings will ensure that your data outputs are valid and usable.
- Display Data in GUIs: If you’re developing applications with user interfaces, formatted strings can help present data more clearly. This makes it easier for users to understand the information displayed.
- Database Queries: Building SQL queries dynamically, especially for reporting, can also benefit from careful string formatting. However, always ensure to use placeholders to prevent SQL injection attacks.
Mastering string formatting can elevate your ability to communicate data effectively. Interested in diving deeper into the subject? You can explore our personalized training options tailor-made for your learning journey. Additionally, our YouTube channel also has a wealth of resources to help you sharpen your programming skills.
Problem 4: Removing Punctuation and Whitespace
Cleaning up strings by removing punctuation and whitespace is a common necessity in data preprocessing. Prioritizing this step can lead to more accurate data analysis and cleaner datasets. Let’s explore effective methods to achieve this and understand the impact of string cleaning on data accuracy.
Methods to Clean Strings:
When it comes to eliminating unwanted characters, punctuation, and excess whitespace in Python, there are several straightforward methods to consider. Here are some commonly used techniques:
- Using the
string
Module: Thestring
module provides a convenient constant calledstring.punctuation
that includes all punctuation characters. You can combine this with list comprehensions or loops to filter out unwanted characters:import string original_string = "Hello, World! This is a test." cleaned_string = ''.join(char for char in original_string if char not in string.punctuation) print(cleaned_string) # Output: Hello World This is a test
- Regular Expressions: For more complex cleaning, Python’s
re
module is extremely useful. You can remove both punctuation and extra whitespace in a single line:import re original_string = "Hello, World! This is a test." cleaned_string = re.sub(r'[^\w\s]', '', original_string) # Removes punctuation cleaned_string = re.sub(r'\s+', ' ', cleaned_string) # Replaces multiple spaces with a single space print(cleaned_string.strip()) # Output: Hello World This is a test.
- The
str.replace()
Method: If you want to target specific punctuation marks, you can use thereplace()
method. Note that this method is less flexible than using regex but can be sufficient for simple cases:original_string = "Hello, World! This is a test." cleaned_string = original_string.replace(',', '').replace('!', '') print(cleaned_string) # Output: Hello World This is a test.
- Using
str.strip()
: Whilestrip()
is often used for trimming leading and trailing whitespace, when combined with others methods, you can clean your strings further. For example, using it after a punctuation cleanup can tidy up any spaces left behind:original_string = " Hello, World! " cleaned_string = ''.join(char for char in original_string if char not in string.punctuation).strip() print(cleaned_string) # Output: Hello World
This combination of methods allows you to deal with most common scenarios you’ll encounter while cleaning strings.
Impact on Data Accuracy:
Cleaning strings isn’t just about aesthetics; it can significantly enhance the accuracy of data analysis. Here’s how cleaning up punctuation and whitespace can help:
- Increased Consistency: Data that is consistently formatted is easier to analyze. Removing unwanted characters ensures uniformity, which is especially crucial when merging datasets or comparing values.
- Improved Searchability: When analyzing textual data, removing punctuation and extra whitespace can help improve search results. Queries become more effective when the strings are homogenized. Think of searching for a term in a messy dataset full of inconsistent punctuation!
- Better Performance: Reducing the complexity of strings by stripping unwanted characters can lead to improved performance in data processing. Less data to parse means faster computation times—all while retaining the integrity of the essential data.
- Data Quality Assurance: String cleaning is a vital component of data quality processes. The clearer the data, the more reliable your insights will be. This is crucial for data engineers who rely on accurate representations in dashboards or reporting tools.
To dive deeper into effective string manipulation, explore our personalized training options. Also, don’t miss out on more practical insights by visiting our YouTube channel for a wealth of coding resources that can enhance your learning experience.
Problem 5: String Encoding and Decoding
String encoding and decoding might sound technical, but understanding it is essential for data engineers, especially as we deal with data in various formats every day. Whether you’re working on data transmission, storage, or simply manipulating strings in your Python project, you need to grasp this concept. Let’s break it down.
Understanding Encoding and Decoding
At its core, string encoding refers to converting characters into a specific format that can be stored or transmitted. In contrast, decoding reverses this process, translating the stored or transmitted data back into a readable format.
Think of it like translating a book into another language. When you encode a string, you’re changing the characters into a different representation (like converting “Hello” to its byte representation). When decoding, you’re translating that representation back into the original string.
In Python, it’s easy to understand how encoding and decoding work. Here’s a straightforward example using UTF-8 encoding, one of the most common encoding standards:
# Encoding a string original_string = "Hello, World!" encoded_string = original_string.encode('utf-8') print(encoded_string) # Output: b'Hello, World!' # Decoding the byte string back to the original decoded_string = encoded_string.decode('utf-8') print(decoded_string) # Output: Hello, World!
In this example, encode()
converts the string into bytes, and decode()
converts it back. Isn’t that simple? Just remember, encoding is crucial when you need to store or transfer text data efficiently.
Understanding encoding is applicable in various real-world scenarios, particularly in handling file formats or network communications where data needs to be in a specific format for correct processing.
Handling Data Transmission
How does string encoding relate to data transmission and storage in data engineering? Well, when you’re transmitting data across networks or storing it in databases, you often need to ensure that your text gets transmitted accurately without errors.
- Data Transmission: When sending data over a network, encoding helps maintain the integrity of the transmitted information. If the byte representation doesn’t match what the receiver expects, it could lead to garbled text or data loss. Ensuring both sender and receiver agree on the encoding format (like UTF-8) is essential.
- Data Governance: As data privacy concerns grow, understanding how your data is encoded becomes crucial. For instance, regulations often require that sensitive customer information is encrypted before transmission. This means encoding it in a secure format, ensuring only authorized parties can decode it.
- Storage Efficiency: Utilizing efficient encoding can save storage space when dealing with massive datasets. For example, when working with large file systems, choosing the right encoding can minimize the amount of data that needs to be stored or retrieved, ultimately improving performance.
- Error Handling: Finally, it’s important to have proper mechanisms in place for handling encoding errors. Unexpected character sequences can cause problems during data processing. Leveraging robust error handling during the encoding and decoding stages ensures your data remains accessible.
Whether you’re preparing data to be sent over APIs or ensuring it’s properly formatted before saving to a database, mastering string encoding and decoding improves your workflow. If you’re eager to learn more about these concepts, consider our personalized training options to enhance your understanding in practical applications. You can also check out our YouTube channel for tutorials and hands-on resources regarding Python programming.
Enhancing Coding Skills with Python Challenges
Boosting your coding skills doesn’t just come from reading books or watching videos. Engaging in hands-on coding challenges is one of the most effective ways to reinforce concepts and build your confidence. Python offers a plethora of challenges that can help you practice string manipulation, which is an essential skill for any data engineer or programming enthusiast. By solving these challenges, you’ll develop a more profound understanding of string operations, recognize patterns, and enhance your problem-solving skills.
Here, we’ll look at some recommended resources to support you on this journey.
Recommended Resources
To boost your coding journey with Python, various resources are available that cater to different learning styles. Explore these tools to refine your skills:
- Data Engineer Academy’s Personalized Training: Our tailored training program focuses on your unique learning path, blending theory with practical exercises. Whether you’re a beginner or looking to sharpen your skills, this program can adapt to meet your needs. Start today with our personalized training.
- Video Tutorials: YouTube is an excellent platform for hands-on learning. Check out the Data Engineer Academy YouTube channel, where you will find a variety of video tutorials. These cover not only Python string manipulation challenges but also extensive topics on data engineering, providing a well-rounded educational experience.
- 100+ Python Coding Questions for Data Engineers: Looking for a more extensive array of challenges? Dive into the 100+ Python Coding Questions for Data Engineers for practice. These questions are specifically designed to help you hone your skills tailored for real-world applications.
- Python vs. R for Data Science: As you explore string challenges, consider how Python compares with R. Our guide on Python vs. R for Data Science provides insights into which language may be better suited for specific tasks.
Each of these resources can enhance your understanding and application of Python, particularly as you tackle string manipulation problems. They will empower you to tackle challenges with a lot more confidence and prepare you for your career in data engineering.
Conclusion
Mastering string manipulation in Python is an essential step toward becoming a more confident and capable programmer. By tackling the challenges we’ve discussed, you not only improve your coding skills but also enhance your ability to handle real-world data scenarios efficiently.
Continuous practice is key. As you refine these skills, don’t hesitate to seek out resources that can support your journey. Our personalized training program is designed to guide you through your learning path, helping you tackle complex concepts with ease.
Remember to stay engaged with the community and explore more insights on our YouTube channel. Keep pushing your boundaries, and enjoy the process of learning and growing as a data engineer!
Real stories of student success
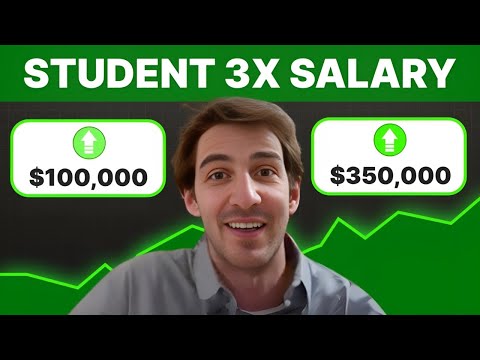
Student TRIPLES Salary with Data Engineer Academy
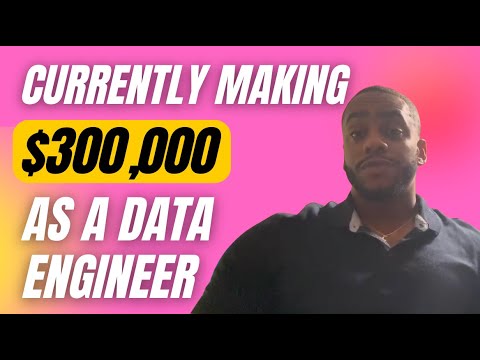
DEA Testimonial – A Client’s Success Story at Data Engineer Academy
Frequently asked questions
Haven’t found what you’re looking for? Contact us at [email protected] — we’re here to help.
What is the Data Engineering Academy?
Data Engineering Academy is created by FAANG data engineers with decades of experience in hiring, managing, and training data engineers at FAANG companies. We know that it can be overwhelming to follow advice from reddit, google, or online certificates, so we’ve condensed everything that you need to learn data engineering while ALSO studying for the DE interview.
What is the curriculum like?
We understand technology is always changing, so learning the fundamentals is the way to go. You will have many interview questions in SQL, Python Algo and Python Dataframes (Pandas). From there, you will also have real life Data modeling and System Design questions. Finally, you will have real world AWS projects where you will get exposure to 30+ tools that are relevant to today’s industry. See here for further details on curriculum
How is DE Academy different from other courses?
DE Academy is not a traditional course, but rather emphasizes practical, hands-on learning experiences. The curriculum of DE Academy is developed in collaboration with industry experts and professionals. We know how to start your data engineering journey while ALSO studying for the job interview. We know it’s best to learn from real world projects that take weeks to complete instead of spending years with masters, certificates, etc.
Do you offer any 1-1 help?
Yes, we provide personal guidance, resume review, negotiation help and much more to go along with your data engineering training to get you to your next goal. If interested, reach out to [email protected]
Does Data Engineering Academy offer certification upon completion?
Yes! But only for our private clients and not for the digital package as our certificate holds value when companies see it on your resume.
What is the best way to learn data engineering?
The best way is to learn from the best data engineering courses while also studying for the data engineer interview.
Is it hard to become a data engineer?
Any transition in life has its challenges, but taking a data engineer online course is easier with the proper guidance from our FAANG coaches.
What are the job prospects for data engineers?
The data engineer job role is growing rapidly, as can be seen by google trends, with an entry level data engineer earning well over the 6-figure mark.
What are some common data engineer interview questions?
SQL and data modeling are the most common, but learning how to ace the SQL portion of the data engineer interview is just as important as learning SQL itself.