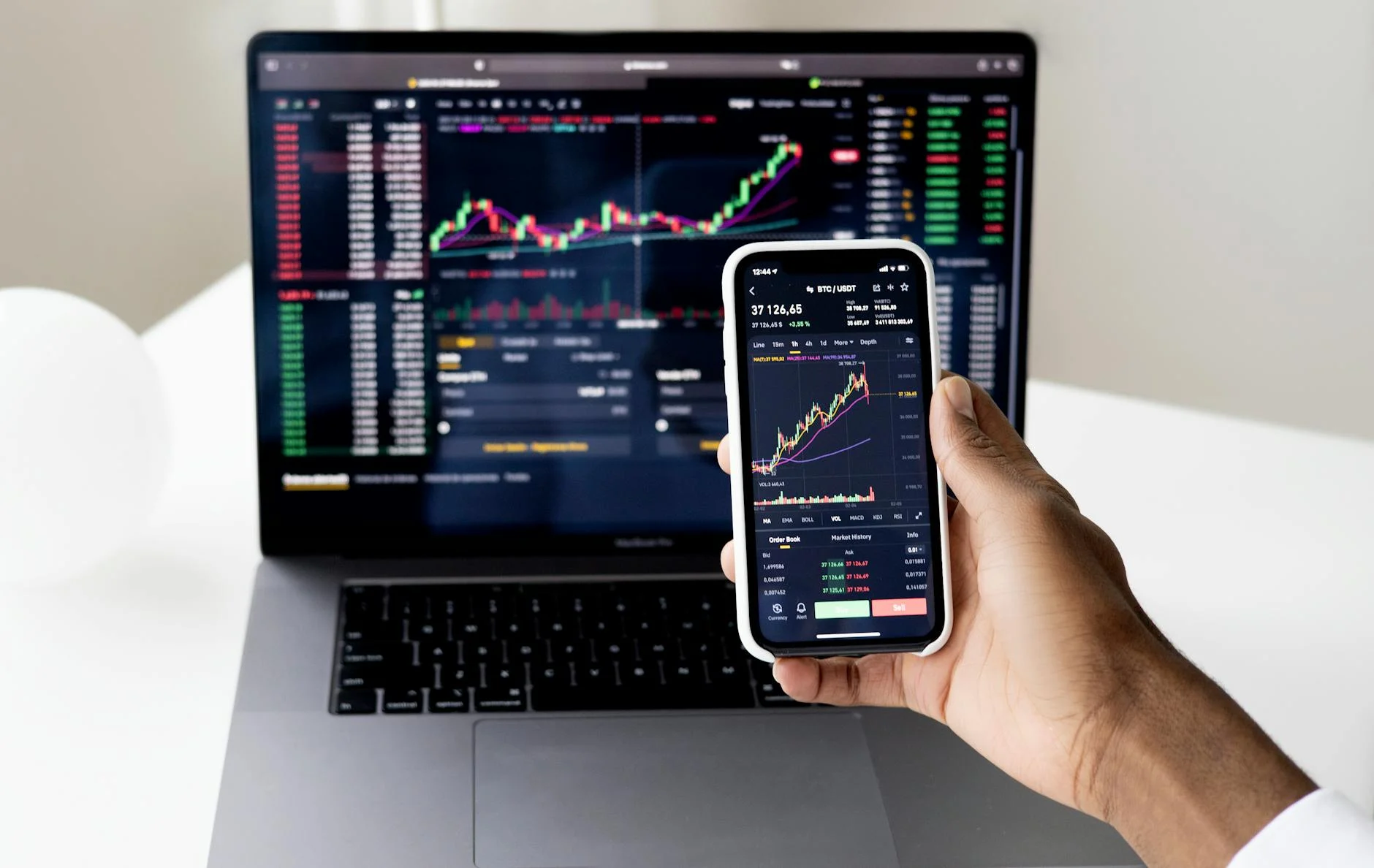
5 Real World Free Projects You Can Start Today
Ever feel stuck trying to put what you’ve learned into action? Hands-on projects are the best way to bridge that gap. They’re not just great for learning — they let you prove your skills, build something meaningful, and even help others along the way. Whether you’re looking to boost your career, grow personally, or contribute to your community, real-world projects give you that edge. And guess what? You don’t need a massive budget or years of experience to get started. Today, we’re talking about five free, impactful projects you can pick up right now. Ready to make a difference while leveling up? Let’s jump in.
Build a Real-Time Chat Application
Creating a real-time chat application is one of the most practical and exciting projects you can dive into as a developer. It’s not just about sending messages — it’s about creating an interactive experience where people can connect instantly with friends, family, or coworkers. Whether for personal use, gaming, or productivity, a well-designed chat app combines speed, reliability, and responsiveness. Let’s break this down into actionable steps to help you get started.
Core Features of a Chat Application
A good chat application goes beyond simple messaging. Here are the core features that make any chat app functional and user-friendly:
- One-on-One Messaging: The backbone of any chat app is the ability to send messages directly between users. This ensures private, real-time conversations.
- Group Chat: Allow users to create or join group conversations to stay connected with a wider circle. Group chat functionality, often with admin controls, adds extra value.
- Instant Notifications: Notify users when new messages arrive, even when they’re not actively in the app. Push notifications provide a seamless experience.
- Typing Indicators: Show users when someone is typing, replicating the natural flow of a face-to-face conversation.
- Message Read Status: Read receipts or delivery indicators let users know their messages have been received or read.
- Security and Privacy: End-to-end encryption is critical for safeguarding user data and maintaining trust.
- File Sharing: Users value the ability to share images, links, or documents directly within the chat.
Every one of these features improves functionality and keeps users engaged. Missing even a few can make an app feel incomplete or clunky.
Technologies and Tools to Use
What powers real-time chat applications? Here’s a brief look at the technologies you’ll need:
- Backend Frameworks:
- Django or Flask: If you’re familiar with Python, these frameworks help you quickly build a robust server-side application.
- Node.js with frameworks like Express can also fit, offering lightweight, event-driven architecture.
- Real-Time Communication Tools:
- WebSockets: Enables two-way communication between a server and clients for real-time updates. Popular libraries include Socket.IO or Django Channels.
- Alternatively, consider using Firebase for integrated real-time database capabilities that scale automatically.
- Frontend Development:
- Pair HTML, CSS, and JavaScript for your core interface development. Frameworks like React or Vue.js make building dynamic UIs easier.
- Prefer mobile-first? Libraries like React Native can help create cross-platform experiences for iOS and Android.
- Database:
- Use PostgreSQL or MongoDB for a scalable solution.
- For simpler use cases, Firebase Realtime Database works well for storing and syncing data in real time.
- Push Notifications: Implement services like OneSignal, Firebase Cloud Messaging, or custom-built solutions to alert users.
By choosing the right technologies and libraries, you can save time on coding while keeping your application future-proof.
Steps to Get Started
Ready to dive in? Let’s simplify the process so you can get started without feeling overwhelmed:
- Set Up Your Environment:
- Install foundational tools like Python, Node.js, or your preferred backend platform.
- Use version control tools (hello, GitHub) to track progress and collaborate if needed.
- Create Your Backend:
- Build the foundational logic for users, authentication, and message handling.
- Set up WebSockets for real-time communication. In Django, for example, the Django Channels library provides WebSocket support.
- Design the UI:
- Use a CSS framework like Tailwind CSS or Bootstrap for responsive design.
- Start simple: focus on a clean chat window, input box, and message threads.
- Add Core Features:
- Integrate real-time messaging using Socket.IO or Firebase.
- Implement chat history by saving messages in your database.
- Test With Scalability in Mind:
- Simulate up to 1,000 users using tools like Apache JMeter to ensure the app remains responsive.
- Deploy:
- Host your backend on Heroku or AWS, and deploy your frontend with platforms like Netlify.
- Troubleshoot and improve based on user feedback—this step is golden for success!
This sequence takes you from setup to deployment without overloading you with complexities. Focus on building your MVP (Minimum Viable Product) first, and improve as you go.
This is the perfect project to show off your technical skills while making something others can actually use. Ready to start coding? Stay tuned for more real-world ideas.
Create a Stock Price Tracker
If you’ve ever wondered how to keep up with the stock market like a pro, this project is your answer. A stock price tracker isn’t just a cool piece of tech—it’s an invaluable tool for following market trends, analyzing investment opportunities, or just staying informed. This project challenges you to integrate real-world financial data while honing your programming and problem-solving skills. Let’s break down what goes into creating one.
Features of a Stock Tracker
What makes a stock tracker useful? Here are the key features your application should include:
- Live Price Updates: Display real-time stock prices for selected companies. This feature ensures users never miss market changes.
- Custom Alerts: Let users set price thresholds. Imagine getting notified when your favorite stock hits a buy or sell mark.
- Historical Data Visualization: Include features for graphically viewing stock price history. Line or candlestick charts can visually present trends over time.
- Multi-Stock Tracking: Allow tracking of multiple stocks simultaneously. Users can maintain a portfolio rather than tracking one stock at a time.
With these features in place, your stock tracker evolves into an all-in-one solution that’s functional and easy to use.
Required APIs and Tools
Building a stock tracker requires some external tools and libraries. Here’s what you’ll need:
- Financial APIs:
- IEX Cloud: Provides real-time stock prices, historical data, and other financial information. It’s reliable and easy to use.
- Alpha Vantage: Another fantastic API offering free plans that include daily pricing and technical data. Perfect for beginners.
- Programming Languages and Libraries:
- Python is a great choice for this project, thanks to its simplicity and rich ecosystem.
- Libraries you’ll want to explore:
- Requests: For making API calls.
- pandas: To process and analyze data.
- Matplotlib or Plotly: For creating dynamic and interactive data visualizations.
- IDE and Hosting Platform:
- Use an IDE like VS Code or PyCharm for efficient coding.
- For deployment, look into Streamlit (great for dashboards) or host on Heroku to share your tracker.
By leveraging powerful APIs and Python libraries, you reduce the complexity and focus on the fun parts of coding.
Implementation Guide
Here’s how you can get your stock price tracker up and running from scratch:
- Set Up Your Environment:
- Install Python if you don’t already have it.
- Install essential Python libraries using
pip install pandas requests matplotlib
(or any others you choose).
- Build the Backend:
- Start with the API integration. Use
requests
to fetch stock price data via endpoints provided by Alpha Vantage or IEX Cloud. - Fetch current prices, historical data, or even news headlines linked to stocks.
- Write logic for alert notifications. This could run periodically using Python’s
schedule
library or a cron job.
- Start with the API integration. Use
- Process the Data:
- Use pandas to clean and process data. For example, convert timestamps or calculate growth percentages.
- Aggregate stock data for easier multi-stock tracking.
- Create Visualizations:
- Use Matplotlib for static graphs (like bar charts or line graphs).
- For interactive elements, go with Plotly to build candlestick graphs or slider-based trends.
- Develop a Frontend Dashboard:
- Keep it simple to start. Use tools like Streamlit for a clean UI and interactive elements.
- Feature a search bar where users can enter stock ticker symbols.
- Embed charts and price stats onto one easy-to-navigate dashboard.
- Test and Launch:
- Test your app with various stock symbols to confirm accuracy.
- Deploy online using services like Streamlit Cloud, Heroku, or AWS.
A polished stock price tracker combines functionality with clean, logical design. Once complete, you’ll have a personal finance tool that showcases your technical prowess. And guess what? You’re also learning skills that are highly valued in any data-centric job market!
Build a Real-Time Language Translator
Want a project that’s both fun and incredibly useful? Building your own real-time language translator isn’t just a great way to learn—it’s the kind of app that has real-world applications. Imagine breaking language barriers on the spot, whether it’s for work, travel, or just everyday conversations. You’ll have the chance to work with cutting-edge APIs, craft a clean interface, and develop an application that creates value.
Features of the Translator
A powerful translator is more than just a word-for-word converter. Here’s what your translator should include to stand out:
- Support for Multiple Languages: Allow users to select from a wide variety of languages. The broader the coverage, the more accessible your tool becomes.
- Text-to-speech conversion: Users can hear translations instead of just reading them. Great for practicing pronunciation or when typing takes too long.
- Concurrent Language Processing: Make translations instant by handling both input and output seamlessly. For example, support real-time exchanges where one user speaks their native language, and the app translates it live for the other user.
These features ensure that your app feels polished and practical while offering functionality people actually need.
APIs and Tools for Development
The hardest part of coding your translator is already done—thanks to APIs. These tools save you from creating everything from scratch while still giving you control. Here are the key tools you’ll need:
- Google Translate API: This is your main translator. It’s incredibly accurate, supports dozens of languages, and offers straightforward integration into your app.
- SpeechRecognition Library: For enabling voice input. This Python library recognizes spoken words and converts them to text.
- gTTS (Google Text-to-Speech): Need text-to-speech functionality? This tool generates lifelike speech for any text output.
- WebSockets: To enable real-time language processing during live conversations, WebSockets allows constant communication between your app and its server.
These services combine ease of use with reliability, giving your translator everything it needs to impress.
Development Guide
Ready to roll up your sleeves? Let’s walk through how to create your translator, breaking it down into manageable steps:
- Set Up Your Environment:
- Install Python and essential libraries, including SpeechRecognition, gTTS, and Flask if you’re building a web app.
- Secure an API key for Google Translate by signing up for a Google Cloud account and enabling the Translate API.
- Create the Backend:
- Use Flask (or your preferred web framework) to create endpoints for translating text.
- Write code that takes text input, calls the Google Translate API, and sends back the translation to the front end.
- Add support for SpeechRecognition input so users can speak instead of typing.
- Add Text-to-Speech Features:
- For every translation, use gTTS to generate an audio file that users can listen to.
- Save the files temporarily or stream them directly for faster access.
- Build the Frontend UI:
- Design an input field for users to type or speak their text.
- Include dropdowns or auto-detect settings for input/output language selection.
- Add a play button next to the translated text for text-to-speech playback.
- Integrate Real-Time Capabilities:
- Use WebSockets for live conversation support. Users can talk in one language while responses are translated instantly.
- Implement a delay buffer for smoother translations in real-time exchanges.
- Test Thoroughly:
- Use common language pairs like English to Spanish, or test harder ones, such as Chinese to French.
- Check text-to-speech clarity, translation accuracy, and the speed of real-time processing.
- Deploy Your Translator:
- Share your work by deploying the backend on platforms like Heroku or AWS.
- Host the frontend on Netlify or Firebase Hosting, making your app available worldwide.
This hands-on project helps you learn API integration, improve your coding logic, and understand real-time communication systems—all with a tangible product you can share with pride.
Conclusion
The best way to grow your skills isn’t by just reading or watching tutorials—it’s by tackling real-world projects that push you out of your comfort zone. The five free projects we discussed are more than learning exercises; they’re opportunities to build something meaningful while leveling up your expertise.
Choose a project that excites you and aligns with your goals. Whether you’re coding a real-time chat app, tracking live stock prices, or creating a food delivery tracker, the key is to start small and keep improving. These hands-on experiences will not only reinforce your knowledge but also give you something tangible to showcase in your portfolio.
Your next step? Don’t overthink it — pick a project, set a deadline, and start building. What you create today could be the foundation for tomorrow’s success. Which project are you drawn to? Share your thoughts, and let’s keep learning together!
Real stories of student success
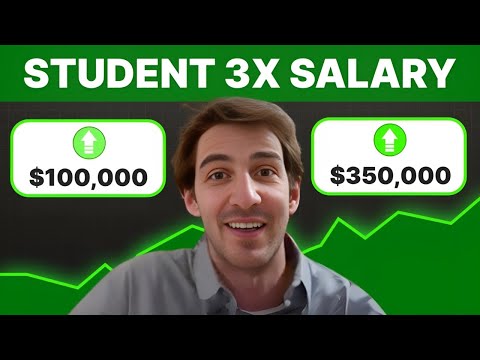
Student TRIPLES Salary with Data Engineer Academy
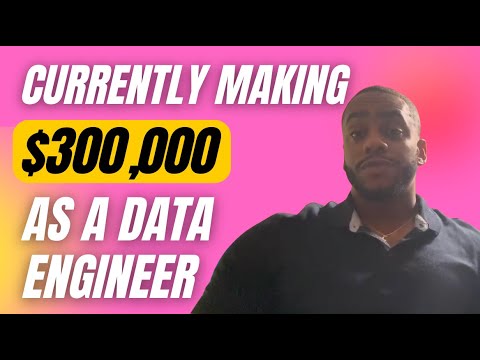
DEA Testimonial – A Client’s Success Story at Data Engineer Academy
Frequently asked questions
Haven’t found what you’re looking for? Contact us at [email protected] — we’re here to help.
What is the Data Engineering Academy?
Data Engineering Academy is created by FAANG data engineers with decades of experience in hiring, managing, and training data engineers at FAANG companies. We know that it can be overwhelming to follow advice from reddit, google, or online certificates, so we’ve condensed everything that you need to learn data engineering while ALSO studying for the DE interview.
What is the curriculum like?
We understand technology is always changing, so learning the fundamentals is the way to go. You will have many interview questions in SQL, Python Algo and Python Dataframes (Pandas). From there, you will also have real life Data modeling and System Design questions. Finally, you will have real world AWS projects where you will get exposure to 30+ tools that are relevant to today’s industry. See here for further details on curriculum
How is DE Academy different from other courses?
DE Academy is not a traditional course, but rather emphasizes practical, hands-on learning experiences. The curriculum of DE Academy is developed in collaboration with industry experts and professionals. We know how to start your data engineering journey while ALSO studying for the job interview. We know it’s best to learn from real world projects that take weeks to complete instead of spending years with masters, certificates, etc.
Do you offer any 1-1 help?
Yes, we provide personal guidance, resume review, negotiation help and much more to go along with your data engineering training to get you to your next goal. If interested, reach out to [email protected]
Does Data Engineering Academy offer certification upon completion?
Yes! But only for our private clients and not for the digital package as our certificate holds value when companies see it on your resume.
What is the best way to learn data engineering?
The best way is to learn from the best data engineering courses while also studying for the data engineer interview.
Is it hard to become a data engineer?
Any transition in life has its challenges, but taking a data engineer online course is easier with the proper guidance from our FAANG coaches.
What are the job prospects for data engineers?
The data engineer job role is growing rapidly, as can be seen by google trends, with an entry level data engineer earning well over the 6-figure mark.
What are some common data engineer interview questions?
SQL and data modeling are the most common, but learning how to ace the SQL portion of the data engineer interview is just as important as learning SQL itself.