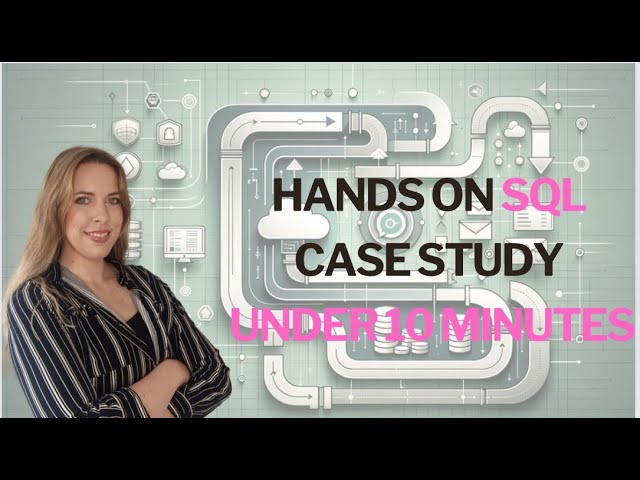
SQL Right Join Explained – Improve Data Integration with Real-World Examples
As data professionals, we’re often tasked with tackling complex, real-world problems that require a deep understanding of SQL and the ability to apply it effectively. In this comprehensive blog post, we’ll dive into an intermediate-level SQL case study that explores practical examples and data analysis techniques to help you enhance your problem-solving skills and make data-driven decisions.
Understanding the Case Statement in SQL
At the heart of this case study lies the SQL `CASE`
statement, a powerful tool that enables us to apply conditional logic to our queries. Unlike the traditional `WHERE`
or `HAVING`
clauses, the `CASE`
statement allows us to create new columns and assign different values based on specific conditions.
The basic syntax for the `CASE`
the statement is as follows:
CASE WHEN condition THEN result WHEN condition THEN result ... ELSE result END
This structure allows us to evaluate multiple conditions and assign corresponding values to a new column. The `ELSE`
clause provides a fallback option for any conditions that are not met by the preceding `WHEN`
statements.
Let’s dive into a few practical examples to better understand the power of the `CASE`
statement:
Example 1: Categorizing Products by ID
Suppose we have a `products`
table that stores information about various products, including their names and category IDs. We want to create a new column called `category`
that assigns a descriptive category name based on the category ID.
SELECT product_name, CASE WHEN category_id = 1 THEN 'Electronics' WHEN category_id = 2 THEN 'Clothing' WHEN category_id = 3 THEN 'Home Decor' ELSE 'Other' END AS category FROM products;
In this example, the `CASE`
statement evaluates the `category_id`
column and assigns a corresponding category name to the new `category`
column. If the `category_id`
does not match any of the specified conditions, the `ELSE`
clause assigns the value ‘Other’.
Example 2: Categorizing Products by Price
Now, let’s consider a scenario where we want to categorize products based on their price. We’ll create a new column called `price_category`
that assigns a label (e.g., ‘Low’, ‘Medium’, ‘High’) based on the product’s unit price.
SELECT product_name, unit_price, CASE WHEN unit_price < 200 THEN 'Low' WHEN unit_price >= 200 AND unit_price <= 500 THEN 'Medium' ELSE 'High' END AS price_category FROM products;
In this example, the `CASE`
statement evaluates the `unit_price`
column and assigns the appropriate price category label to the new `price_category`
column. If the price is less than 200, it’s categorized as ‘Low’; if the price is between 200 and 500, it’s categorized as ‘Medium’; and if the price is greater than 500, it’s categorized as ‘High’.
Applying the Case Statement to a Real-World Scenario
Now that we’ve covered the basics of the `CASE`
statement, let’s dive into a more complex, real-world scenario and see how we can apply these techniques to solve a practical problem.
The Challenge
Imagine you’re working for an e-commerce company that sells a variety of products. The product team has requested a report that retrieves the product name, unit price, and a new column called `price_category`
that categorizes products into three groups: ‘Low’, ‘Medium’, and ‘High’ based on their price ranges.
The criteria for the price categories are as follows:
- Low: Unit price less than $200
- Medium: Unit price between $200 and $500 (inclusive)
- High: Unit price greater than $500
Solving the Problem with SQL
To solve this problem, we’ll use the `CASE`
statement to create the new `price_category`
column based on the given criteria. Here’s the SQL query:
SELECT product_name, unit_price, CASE WHEN unit_price < 200 THEN 'Low' WHEN unit_price >= 200 AND unit_price <= 500 THEN 'Medium' ELSE 'High' END AS price_category FROM products;
Let’s break down the query step by step:
- The
`SELECT`
statement retrieves the`product_name`
and`unit_price`
columns from the`products`
table. - The
`CASE`
statement evaluates the`unit_price`
column and assigns the appropriate price category label to the new`price_category`
column. - If the
`unit_price`
is less than 200, the`price_category`
is assigned the value ‘Low’. - If the
`unit_price`
is between 200 and 500 (inclusive), the`price_category`
is assigned the value ‘Medium’. - If the
`unit_price`
is greater than 500, the`price_category`
is assigned the value ‘High’.
By using the `CASE`
statement, we’ve created a new column that categorizes the products based on their price, making it easier for the product team to analyze and understand the pricing distribution of the products.
Enhancing the Analysis with Additional Techniques
While the previous solution solves the immediate problem, there are additional techniques we can explore to further enhance the analysis and provide more insights to the product team.
Calculating Price Range Statistics
In addition to the price category, the product team might be interested in understanding the overall price distribution of the products. We can calculate some basic statistics, such as the minimum, maximum, and average prices, to provide a more comprehensive view of the product pricing.
SELECT MIN(unit_price) AS min_price, MAX(unit_price) AS max_price, AVG(unit_price) AS avg_price, CASE WHEN unit_price < 200 THEN 'Low' WHEN unit_price >= 200 AND unit_price <= 500 THEN 'Medium' ELSE 'High' END AS price_category, COUNT(*) AS product_count FROM products GROUP BY price_category ORDER BY price_category;
This query not only includes the `price_category`
column but also calculates the minimum, maximum, and average prices for each category. Additionally, it provides the count of products in each price category, giving the product team a better understanding of the overall product pricing distribution.
Visualizing the Data
To further enhance the analysis, you could consider integrating the SQL results with a data visualization tool, such as Tableau or Power BI. By creating interactive dashboards and charts, you can help the product team better understand the pricing trends and make more informed decisions.
For example, you could create a bar chart or a pie chart to visualize the distribution of products across the different price categories. This would provide a clear, visual representation of the pricing landscape, making it easier for the product team to identify any potential pricing gaps or opportunities.
Applying SQL Skills to Real-World Challenges
The case study we’ve explored in this blog post demonstrates the importance of having a strong understanding of SQL and the ability to apply it to real-world scenarios. By mastering techniques like the `CASE`
statement, you can tackle complex problems, extract valuable insights from data, and support data-driven decision-making within your organization.
The Data Engineer Academy offers a range of courses and learning materials to help you become a data engineering expert.
Remember, the key to success in data engineering and SQL is continuous learning, practice, and the ability to apply your skills to real-world problems. By embracing this mindset, you’ll be well on your way to becoming a data analysis and problem-solving expert.
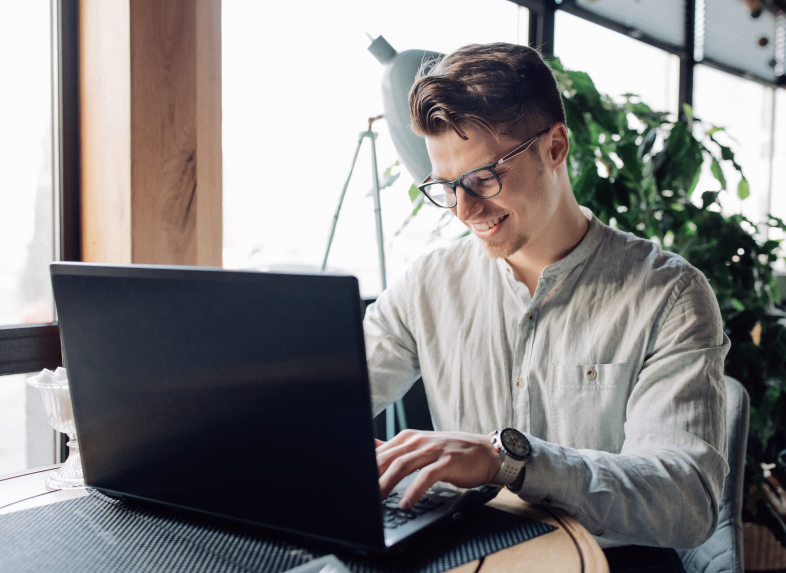
Unlock Your Career Potential
Upskill and start shaping your future with DEAcademy today.
Frequently asked questions
Haven’t found what you’re looking for? Contact us at [email protected] — we’re here to help.
What is the Data Engineering Academy?
Data Engineering Academy is created by FAANG data engineers with decades of experience in hiring, managing, and training data engineers at FAANG companies. We know that it can be overwhelming to follow advice from reddit, google, or online certificates, so we’ve condensed everything that you need to learn data engineering while ALSO studying for the DE interview.
What is the curriculum like?
We understand technology is always changing, so learning the fundamentals is the way to go. You will have many interview questions in SQL, Python Algo and Python Dataframes (Pandas). From there, you will also have real life Data modeling and System Design questions. Finally, you will have real world AWS projects where you will get exposure to 30+ tools that are relevant to today’s industry. See here for further details on curriculum
How is DE Academy different from other courses?
DE Academy is not a traditional course, but rather emphasizes practical, hands-on learning experiences. The curriculum of DE Academy is developed in collaboration with industry experts and professionals. We know how to start your data engineering journey while ALSO studying for the job interview. We know it’s best to learn from real world projects that take weeks to complete instead of spending years with masters, certificates, etc.
Do you offer any 1-1 help?
Yes, we provide personal guidance, resume review, negotiation help and much more to go along with your data engineering training to get you to your next goal. If interested, reach out to [email protected]
Does Data Engineering Academy offer certification upon completion?
Yes! But only for our private clients and not for the digital package as our certificate holds value when companies see it on your resume.
What is the best way to learn data engineering?
The best way is to learn from the best data engineering courses while also studying for the data engineer interview.
Is it hard to become a data engineer?
Any transition in life has its challenges, but taking a data engineer online course is easier with the proper guidance from our FAANG coaches.
What are the job prospects for data engineers?
The data engineer job role is growing rapidly, as can be seen by google trends, with an entry level data engineer earning well over the 6-figure mark.
What are some common data engineer interview questions?
SQL and data modeling are the most common, but learning how to ace the SQL portion of the data engineer interview is just as important as learning SQL itself.