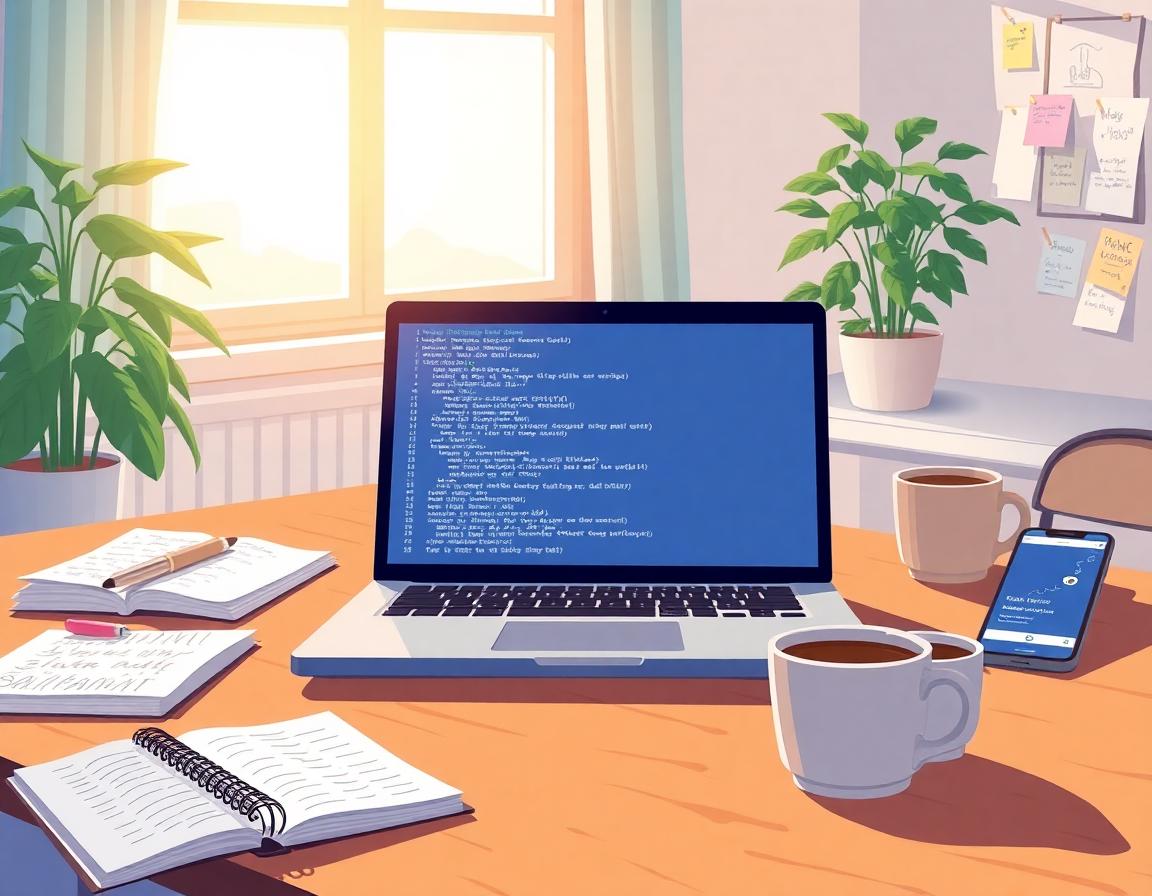
Everyday Python: Practical Coding Questions Based on Real-World Examples
Have you ever wondered how Python can transform everyday tasks into efficient workflows? For data engineers and career shifters, mastering practical coding skills isn’t just a bonus—it’s essential. This post takes a hands-on approach, presenting coding questions based on real-world scenarios to sharpen your understanding and application of Python in daily tasks.
We’ll tackle various coding challenges that simulate the types of problems you might face in a professional environment. Whether you’re prepping for an interview or just looking to enhance your skills, these examples will help you think critically and solve problems like a pro. Plus, if you want personalized guidance tailored to your career goals, check out our personalized training options.
As you navigate through these practical coding questions, don’t forget to explore our YouTube channel for more tips and insights. Let’s jump into the world of Python and discover how you can elevate your coding game today.
Practical Coding Challenges in Data Engineering
Mastering practical coding challenges in data engineering is crucial for enhancing your skills and preparing for real-world scenarios. Here’s a look into key areas where Python shines, making these tasks manageable and efficient.
Data Manipulation Tasks
Data cleaning and transformation are essential in any data engineering role. For instance, consider a CSV file with messy data—missing values, inconsistent formats, and duplicates. You can put Python to work using libraries like Pandas to tackle these issues effectively:
- Cleaning Data: Suppose you have a dataset of customer information. You can use Pandas to identify and fill missing values:
import pandas as pd df = pd.read_csv('customers.csv') df['email'].fillna('[email protected]', inplace=True)
- Transforming Data: Imagine needing to convert a string column into datetime for analysis. With Python, it’s a breeze:
df['signup_date'] = pd.to_datetime(df['signup_date'])
- Removing Duplicates: When dealing with duplicate entries, simply drop them with:
df.drop_duplicates(inplace=True)
These tasks illustrate how Python can streamline data manipulation processes, making it easier to prepare datasets for analysis or reporting. If you’re interested in more about the tools available, check out our blog on Data Engineering Tools in 2023.
Automating Data Workflows
Efficiency is key in data engineering, and Python excels in automating repetitive tasks. From scheduling data loads to orchestrating complex pipelines, Python provides flexible solutions. For example, you might use the schedule
library to run jobs daily:
import schedule import time def job(): print("Running data pipeline...") schedule.every().day.at("10:30").do(job) while True: schedule.run_pending() time.sleep(1)
This straightforward setup could save hours each week, allowing you to focus on higher-value tasks. Explore how you can automate processes further in our article on Data Orchestration: Process and Benefits.
Handling Big Data with Python
When it comes to big data, Python doesn’t fall short. Libraries such as Dask and PySpark enable data engineers to work with massive datasets effortlessly. For instance, Dask extends Pandas capabilities by leveraging parallel computing, which can be crucial for performance:
import dask.dataframe as dd dask_df = dd.read_csv('large_dataset.csv') mean_value = dask_df['col'].mean().compute()
In this example, Dask handles a large dataset without overwhelming your system’s memory. For a deeper dive into big data handling with Python, take a look at our PySpark tutorial for beginners.
Mastering these coding challenges not only enhances your capabilities but also makes you more marketable as a data engineer. If you’re looking for personalized training to boost your skills, consider our personalized training programs. Don’t forget to check out our YouTube channel for more practical examples and tips!
Real-World Example Projects
When it comes to applying Python in practical scenarios, real-world example projects can be your best teachers. They bridge theory with practical application, showing you how to solve genuine challenges you may encounter as a data engineer. Let’s take a deeper look into some impactful projects that illustrate Python’s usefulness.
Building Data Pipelines
Creating robust data pipelines is a central task for many data engineers. A well-constructed pipeline ensures seamless data flow from source to destination. Here’s a quick outline of the steps involved:
- Define Requirements: Start by identifying what data you need and how fresh it needs to be. This helps shape the structure of your pipeline.
- Select the Right Tools: Utilize libraries like Pandas, Airflow, or Prefect to assist in building your pipeline. Python’s versatility allows you to mix different tools as needed.
- Set Up Data Ingestion: Implement mechanisms to pull data from various sources, like APIs or databases. For instance:
import requests response = requests.get('https://api.example.com/data') data = response.json() # Load the data as a JSON object
- Transform Data: Clean and prepare your data using Python libraries:
import pandas as pd df = pd.DataFrame(data) df.dropna(inplace=True) # Remove null entries
- Load Data: Finally, load your data into a database or data warehouse using connectors:
from sqlalchemy import create_engine engine = create_engine('mysql://username:password@localhost/dbname') df.to_sql('table', engine, if_exists='replace', index=False)
By following these steps, you can build a resilient data pipeline that adapts to your organization’s needs.
SQL Integration with Python
Using SQL queries within Python scripts can significantly enhance your data integration capabilities. This collaboration allows you to engage with databases directly from your code—no need to switch contexts. Here’s how to do it effectively:
- Establish Database Connection: Use SQLAlchemy or the built-in
sqlite3
library for database connections. For example:import sqlite3 conn = sqlite3.connect('example.db') cursor = conn.cursor()
- Execute SQL Queries: You can run complex SQL queries right within your Python scripts. This approach eliminates the need for repetitive code:
cursor.execute("SELECT * FROM users WHERE signup_date > '2023-01-01'") results = cursor.fetchall() print(results)
- Integrate with Dataframe: Combine SQL outputs with Pandas for streamlined data manipulation:
import pandas as pd df = pd.read_sql_query("SELECT * FROM sales", conn)
For a hands-on approach to mastering SQL while integrating with Python, check out SQL Projects for Data Analysts and Engineers: Practical Examples and Best Practices. This resource covers various projects specifically designed to improve your skills.
Case Study: Analyzing Trends
Let’s consider a case study that effectively showcases Python’s power in analyzing trends within large datasets. Suppose a retail company wants to examine sales trends over the past year to strategize for future growth. Here’s how Python comes into play:
- Data Collection: Gather historical sales data, which might include various parameters such as date, sales amount, and product category.
- Data Processing: Use Pandas to clean and transform this data. This step might involve:
import pandas as pd df = pd.read_csv('sales_data.csv') df['date'] = pd.to_datetime(df['date']) df.set_index('date', inplace=True)
- Visualization: Applying libraries like Matplotlib or Seaborn allows for compelling visual insights:
import matplotlib.pyplot as plt df.resample('M').sum()['sales_amount'].plot() # Group by month and sum sales plt.title('Monthly Sales Trend') plt.show()
- Insights and Actions: By analyzing the trends depicted in the visualization, the team identifies peak sales months and adjusts inventory and marketing strategies accordingly.
This case illustrates the power of Python in extracting valuable insights from data, empowering your decision-making processes. If you’re looking to advance your skills further, consider our personalized training options tailored to your learning needs. And remember, for more practical examples, check out our YouTube channel.
Python Coding Questions and Interviews
Preparing for a Python coding interview can feel daunting, but with the right approach, you can build confidence and showcase your skills effectively. Here’s a look at common Python interview questions and practical scenarios to refine your skills.
Common Python Interview Questions
Many interviewers focus on specific Python concepts to gauge your familiarity with the language and your problem-solving skills. Here are some common questions you might encounter, along with a brief explanation of how to approach the answers:
- How do you handle missing data in a DataFrame in Python?
- You can use Pandas to check for and fill missing values efficiently. This might involve using methods like
fillna()
to replace missing data with a default value ordropna()
to remove incomplete entries.
- You can use Pandas to check for and fill missing values efficiently. This might involve using methods like
- Can you explain the difference between deep copy and shallow copy?
- A shallow copy creates a new object but inserts references into it to the objects found in the original. A deep copy, on the other hand, replicates all levels of the original object. This distinction is essential for understanding how data can be altered unintentionally.
- What is list comprehension in Python?
- This feature allows you to create lists with less code. For instance, you can generate a new list by iterating over an existing one:
squares = [x**2 for x in range(10)]
- List comprehensions can make your code cleaner and more efficient.
- This feature allows you to create lists with less code. For instance, you can generate a new list by iterating over an existing one:
These questions are just the tip of the iceberg. To dive deeper into this topic and explore more challenging interviews, check out our Data Engineer Interview Questions With Python [+detailed answers] resource for comprehensive guidance.
Practical Scenarios for Testing Skills
Practical scenarios are invaluable when preparing for an interview—they reflect real-world challenges and help solidify your understanding. Here are a few suggestions to get started:
- Build a Data Cleaning Script: Take a messy dataset, implement cleaning techniques using Pandas, and analyze how effective your solutions are.
- Create a Simple REST API: Use Flask to build a RESTful web service that serves data. This will not only enhance your Python skills but also familiarize you with frameworks used in the industry.
- Automate a Daily Task: Think about a repetitive task you handle in your current job or daily life. Write a Python script that automates this process. For example, you might automate sending emails or compiling reports.
- Work on Mini-Projects: Engage in mini-projects like building a web scraper or a data visualizer with Matplotlib and Pandas. This approach not only solidifies your knowledge but adds weight to your portfolio.
These practical exercises encourage you to apply what you’ve learned, making your preparation far more effective. Tailoring your practice to scenarios that mimic real interview questions will give you a significant edge.
Mastering Python coding questions and practical coding scenarios is essential in building your confidence and prowess as a data engineer. If you want personalized support to further enhance your skills, consider our personalized training. And for more tips and insights, don’t forget to check out our YouTube channel.
Resources for Further Learning
As you progress on your journey with Python, it’s essential to supplement your self-study with structured resources. Here are two excellent avenues to explore that can enhance your coding skills and keep you motivated.
Online Courses and Tutorials
Structured learning paths can help you grasp Python more effectively. Online courses provide a roadmap to guide you through the complexities of the language, allowing you to focus on specific skills relevant to your career goals. Personalized training options can cater to your unique learning needs, ensuring you get the most from your education. Whether you’re just starting or looking to advance your skills, platforms like Data Engineer Academy offer tailored mentorship to deepen your understanding.
Consider enrolling in courses that emphasize hands-on projects. Applying your knowledge in practical scenarios reinforces your learning. When you can connect the dots between theory and practice, you’re more likely to retain the information.
YouTube Channels and Tutorials
Visual learning can be incredibly effective, and YouTube is a treasure trove of coding tutorials. Channels like Data Engineer Academy provide high-quality video content that breaks down complex topics into bite-sized, digestible pieces. These tutorials cover everything from basic coding concepts to advanced data engineering challenges, allowing you to learn at your own pace.
Engaging with video tutorials enables you to see coding in action. You can pause, rewind, and rewatch segments, ensuring you fully understand each concept before moving on. Plus, many videos feature real-world examples that can inspire your projects and career trajectory.
Tap into these resources for a comprehensive learning experience. By combining structured courses with engaging video content, you’ll build a solid foundation in Python that can serve you for years to come.
Conclusion
Mastering Python for practical tasks is essential for anyone in data engineering. This language not only simplifies complex data workflows but also enhances your ability to solve real-world problems effectively. Continuous learning is crucial—by engaging with real-world coding challenges and hands-on projects, you can elevate your skills and stay relevant in the ever-evolving tech landscape.
If you’re seeking tailored support, consider our personalized training options designed to fit your career goals.
And don’t forget to tap into the wealth of knowledge available on our YouTube channel for more insights and practical coding examples. Keep pushing your boundaries—your journey in Python is just beginning!
Real stories of student success
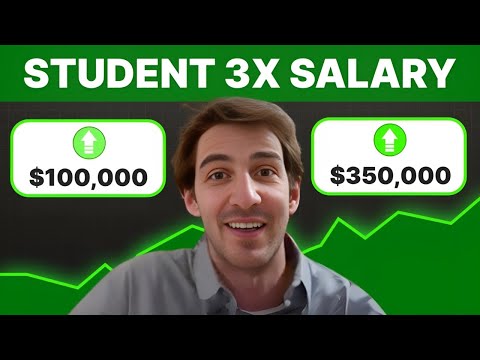
Student TRIPLES Salary with Data Engineer Academy
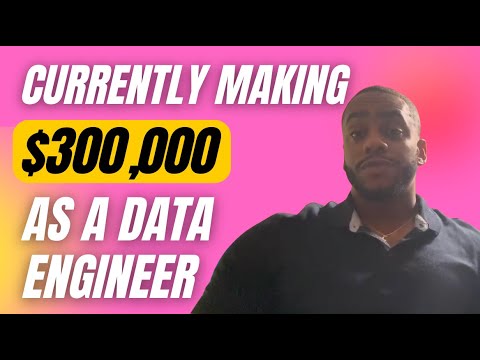
DEA Testimonial – A Client’s Success Story at Data Engineer Academy
Frequently asked questions
Haven’t found what you’re looking for? Contact us at [email protected] — we’re here to help.
What is the Data Engineering Academy?
Data Engineering Academy is created by FAANG data engineers with decades of experience in hiring, managing, and training data engineers at FAANG companies. We know that it can be overwhelming to follow advice from reddit, google, or online certificates, so we’ve condensed everything that you need to learn data engineering while ALSO studying for the DE interview.
What is the curriculum like?
We understand technology is always changing, so learning the fundamentals is the way to go. You will have many interview questions in SQL, Python Algo and Python Dataframes (Pandas). From there, you will also have real life Data modeling and System Design questions. Finally, you will have real world AWS projects where you will get exposure to 30+ tools that are relevant to today’s industry. See here for further details on curriculum
How is DE Academy different from other courses?
DE Academy is not a traditional course, but rather emphasizes practical, hands-on learning experiences. The curriculum of DE Academy is developed in collaboration with industry experts and professionals. We know how to start your data engineering journey while ALSO studying for the job interview. We know it’s best to learn from real world projects that take weeks to complete instead of spending years with masters, certificates, etc.
Do you offer any 1-1 help?
Yes, we provide personal guidance, resume review, negotiation help and much more to go along with your data engineering training to get you to your next goal. If interested, reach out to [email protected]
Does Data Engineering Academy offer certification upon completion?
Yes! But only for our private clients and not for the digital package as our certificate holds value when companies see it on your resume.
What is the best way to learn data engineering?
The best way is to learn from the best data engineering courses while also studying for the data engineer interview.
Is it hard to become a data engineer?
Any transition in life has its challenges, but taking a data engineer online course is easier with the proper guidance from our FAANG coaches.
What are the job prospects for data engineers?
The data engineer job role is growing rapidly, as can be seen by google trends, with an entry level data engineer earning well over the 6-figure mark.
What are some common data engineer interview questions?
SQL and data modeling are the most common, but learning how to ace the SQL portion of the data engineer interview is just as important as learning SQL itself.