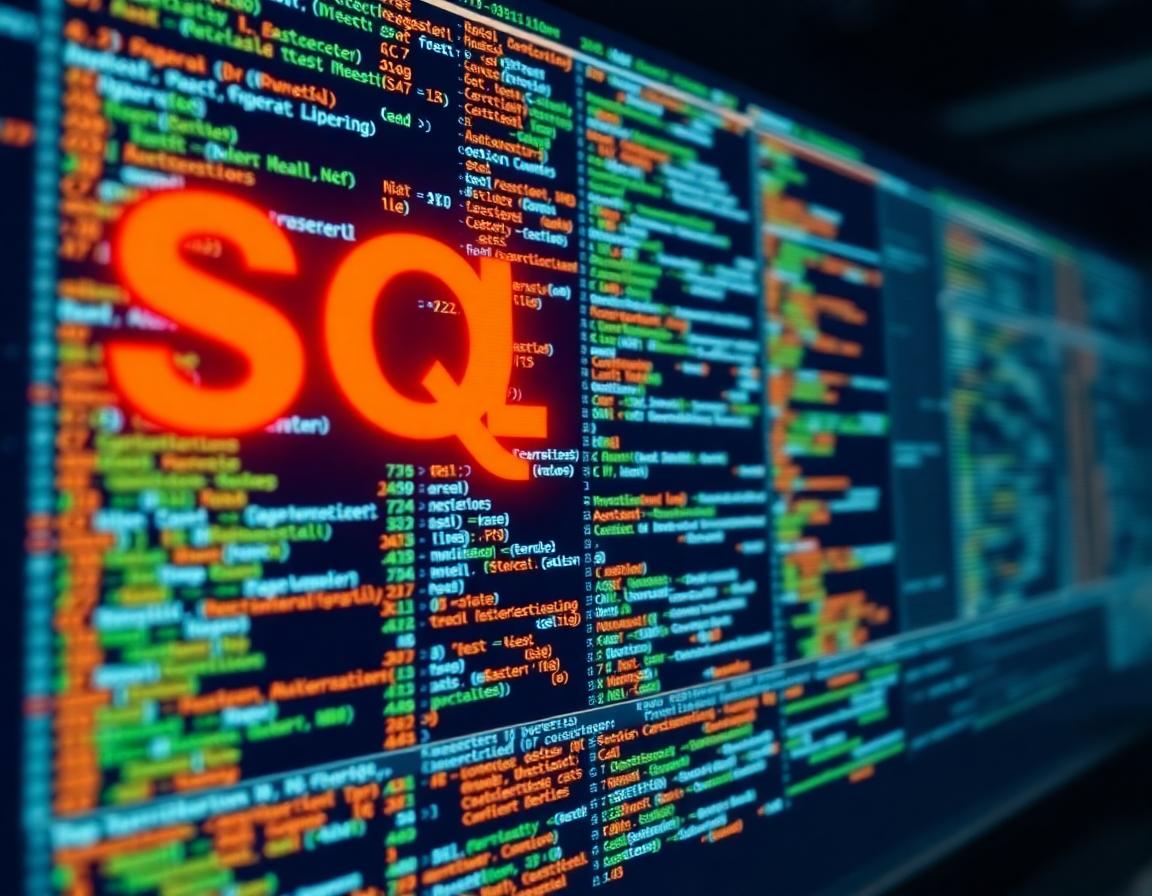
The Best SQL Queries Every Data Engineer Should Know
SQL is a fundamental tool for data engineers, essential for data manipulation and analysis. Knowing the right SQL queries can not only streamline your work but also enhance your ability to extract insights from data. Whether you’re a seasoned data engineer or shifting careers, mastering these queries is key to your success.
In this post, we’ll explore the best SQL queries that every data engineer should be familiar with. You’ll learn not only how to write these queries but also when to apply them for maximum impact. The right SQL skills can open doors, making you more effective in your role.
For those looking to improve their SQL knowledge, consider personalized training at Data Engineer Academy. Additionally, check out other resources like the Data Engineer Academy YouTube channel for practical tips and examples.
Let’s get started on helping you become proficient in the SQL queries that matter most.
Essential SQL Queries Every Data Engineer Should Know
Understanding the core SQL queries is essential for every data engineer. These queries are the building blocks for data manipulation, allowing you to retrieve, modify, and manage data effectively. Let’s break down some of the most critical SQL queries every data engineer should master.
SELECT Statements
SELECT statements are fundamental to SQL. They allow you to retrieve data from one or more tables in a database. By using SELECT, you can specify which columns to return, filter results using the WHERE clause, and order them as needed.
Here’s an example:
SELECT name, age FROM users WHERE age > 18 ORDER BY age;
In this case, the query retrieves the names and ages of users older than 18, sorting them by age. Being comfortable with SELECT statements is crucial as they form the basis for more complex queries and data analysis operations.
JOIN Operations
JOIN operations are essential for combining rows from two or more tables based on a related column. There are several types of JOINs:
- INNER JOIN: Returns records with matching values in both tables.
- LEFT JOIN: Returns all records from the left table and matched records from the right table.
- RIGHT JOIN: Returns all records from the right table and matched records from the left table.
- FULL OUTER JOIN: Returns all records when there is a match in either left or right table records.
For example, to combine user information with their orders:
SELECT users.name, orders.product FROM users INNER JOIN orders ON users.id = orders.user_id;
JOIN operations are vital for creating comprehensive reports and insights from multiple tables.
GROUP BY and Aggregate Functions
The GROUP BY clause is used in conjunction with aggregate functions like COUNT, SUM, and AVG to group rows sharing a property so that aggregate functions can be applied to each group.
For instance:
SELECT user_id, COUNT(order_id) as total_orders FROM orders GROUP BY user_id;
This query summarizes the total number of orders per user. Mastering GROUP BY helps in generating meaningful statistical reports and understanding your data’s trends.
Need more information on using GROUP BY effectively? Check out the GROUP BY SQL Guide.
Subqueries and Common Table Expressions (CTEs)
Subqueries and Common Table Expressions (CTEs) allow for more complex queries. A subquery is a query nested inside another query. For example:
SELECT name FROM users WHERE id IN (SELECT user_id FROM orders WHERE product = 'Gadget');
Here, the subquery finds all user IDs who have ordered a specific product.
A CTE simplifies complex queries and improves readability. It’s defined using the WITH clause. For example:
WITH OrderCount AS ( SELECT user_id, COUNT(order_id) AS cnt FROM orders GROUP BY user_id ) SELECT users.name, OrderCount.cnt FROM users JOIN OrderCount ON users.id = OrderCount.user_id;
Both subqueries and CTEs are handy for breaking down complex logic into manageable parts, making your SQL code cleaner and more understandable.
INSERT, UPDATE, and DELETE Statements
Manipulating data in SQL is done using INSERT, UPDATE, and DELETE statements.
- INSERT: Adds new rows to a table.
INSERT INTO users (name, age) VALUES ('John Doe', 30);
- UPDATE: Modifies existing records.
UPDATE users SET age = 31 WHERE name = 'John Doe';
- DELETE: Removes records from a table.
DELETE FROM users WHERE name = 'John Doe';
Understanding these commands is crucial as they directly affect your data’s integrity and accuracy. For further guidance on data manipulation techniques, consider exploring Data Engineer Academy’s personalized training.
Mastering these essential SQL queries will give you the confidence to tackle your data engineering tasks efficiently. Don’t forget to check out the Data Engineer Academy YouTube channel for practical examples and tips to continue growing your SQL skills.
Advanced SQL Queries for Data Engineering
Mastering advanced SQL queries can significantly enhance the capabilities of data engineers. Knowing how to implement window functions, recursive queries, and control transactions will help you write efficient, powerful SQL code. Here’s a closer look at these advanced topics and how they can be effectively applied in your work.
Window Functions
Window functions are a powerful feature in SQL, enabling you to perform calculations across a set of table rows related to the current row. Unlike regular aggregate functions that summarize data across multiple rows, window functions provide detailed insights without collapsing your result set.
For example, if you want to calculate the running total of sales per user, you can use a window function like this:
SELECT user_id, order_date, amount, SUM(amount) OVER (PARTITION BY user_id ORDER BY order_date) AS running_total FROM orders;
In this query, the SUM
function computes a running total of amount
for each user, ordered by order_date
. The PARTITION BY
clause creates a separate window for each user_id
, allowing you to analyze data more granularly. Window functions excel in scenarios like ranking records, calculating moving averages, or comparing values within a set.
For more insights into using advanced SQL features, check out examples from the Walmart Advance SQL Questions and Costco Advance SQL Question.
Recursive Queries
Recursive queries in SQL enable you to perform operations that require multiple iterations or hierarchical data retrieval. These are particularly useful for dealing with complex data structures, such as organizational charts or bill of materials.
Here’s a basic example using a Common Table Expression (CTE) to retrieve a hierarchy of employees from a table:
WITH RECURSIVE EmployeeCTE AS ( SELECT id, name, manager_id FROM employees WHERE manager_id IS NULL UNION ALL SELECT e.id, e.name, e.manager_id FROM employees e INNER JOIN EmployeeCTE cte ON e.manager_id = cte.id ) SELECT * FROM EmployeeCTE;
In this case, the CTE starts with employees who have no managers (top level), then recursively selects employees reporting to those managers. Use cases for recursive queries include traversing trees or graphs, generating parent-child relationships, or creating cumulative reports.
Transaction Control and Error Handling
Transaction control in SQL ensures that a series of operations are treated as a single unit. This is crucial for maintaining data integrity, especially in cases where an error might occur. The key commands related to transaction control are BEGIN
, COMMIT
, and ROLLBACK
.
Consider this scenario:
BEGIN; UPDATE account SET balance = balance - 100 WHERE user_id = 1; UPDATE account SET balance = balance + 100 WHERE user_id = 2; COMMIT;
Here, either both updates happen, or neither do if something fails (like insufficient balance). If an error occurs, you can roll back the transaction:
ROLLBACK;
Error handling is equally important. Use constructs like TRY...CATCH
in SQL Server or PL/pgSQL for PostgreSQL to catch errors during execution:
BEGIN -- SQL commands here EXCEPTION WHEN others THEN ROLLBACK; -- Handle error END;
Proper transaction control and error handling not only protect the integrity of your data but also give you a robust mechanism for managing complex operations.
For advanced techniques on error handling, explore the article on Using PL/pgSQL: Tips, Tricks, and Common Patterns.
Understanding these advanced SQL queries will elevate your skills as a data engineer, enabling you to handle complex data sets efficiently. For personalized training to deepen your SQL knowledge, consider Data Engineer Academy’s personalized training. Don’t forget to check the Data Engineer Academy YouTube channel for practical examples and further insights!
Performance Optimization Techniques in SQL
Performance optimization is key for efficient SQL queries. As data engineers, you’ll often encounter challenges in making your queries run faster and more efficiently. Here are some proven strategies to keep your SQL performance at its best.
Indexing Strategies
Indexes are like a roadmap for your database. They help database engines find data quickly without searching every row in a table. When you create an index on a column, the database builds a separate data structure to speed up queries that filter or sort on that column.
Different types of indexes serve different purposes:
- Single-column Index: Best for queries that filter on a single column.
- Composite Index: Use this for queries that filter on multiple columns. This can substantially improve performance.
- Unique Index: Ensures that all values in a column are different. It improves search speed and enforces uniqueness.
- Full-text Index: Ideal for searching large text fields. This allows you to search through the text within the column.
Consider using indexes on columns frequently used in WHERE clauses, JOIN conditions, and ORDER BY clauses. However, it’s important to remember that while indexes speed up read operations, they can slow down write operations. Make sure to strike the right balance.
For more on performance optimization techniques, check out the SQL Performance Tuning article.
Query Refactoring
Refactoring your SQL queries can significantly enhance efficiency. This involves rewriting your SQL code to improve its structure without changing the output. An optimized query runs faster, making your database interactions more efficient.
Here are some tips for effective query refactoring:
- Select only needed columns: Instead of using
SELECT *
, specify only the columns you really need. This reduces the amount of data processed. - Eliminate unnecessary subqueries: If possible, use JOINs instead of subqueries, which can lead to better performance.
- Use Common Table Expressions (CTEs): CTEs can simplify complex queries and improve readability.
- Avoid DISTINCT when possible: If you can eliminate duplicate records through proper JOINs or WHERE clauses, it reduces processing time.
Refactoring not only improves performance but also enhances the clarity and maintainability of your SQL code.
Analyzing Query Performance
To optimize your SQL queries effectively, you’ll need to analyze their performance. Several tools and techniques can help you identify slow-running queries and areas for improvement:
- Execution Plans: Most database systems provide access to execution plans. An execution plan shows how a query is executed, detailing the steps and resources used. Use this tool to find bottlenecks in your queries.
- Database Profiling Tools: Tools like SQL Profiler (for SQL Server) or EXPLAIN (for MySQL and PostgreSQL) can help you track query performance over time and find the slowest ones.
- Monitoring Tools: Consider using tools like New Relic or Prometheus to monitor database performance metrics. These can alert you to potential issues before they become problematic.
By actively analyzing query performance, you gain insights into how to tweak and improve your SQL interactions.
For personalized training in SQL optimization techniques, check out Data Engineer Academy’s personalized training. Don’t overlook the value of practical tips available on the Data Engineer Academy YouTube channel to enhance your skills in real-time scenarios.
Practical Applications and Real-World Examples
SQL queries play a crucial role in various data engineering tasks. To understand their importance, let’s look at two real-world case studies: building a data pipeline and executing data migration.
Case Study: Building a Data Pipeline
Creating a data pipeline is essential for any organization that deals with large data sets. A data pipeline automates the movement of data between systems, ensuring it is cleaned, transformed, and stored appropriately.
Consider a scenario where a retail company wants to analyze customer shopping patterns through their sales data. The team uses SQL queries to set up a data pipeline that processes information from various sources like point-of-sale systems, online orders, and customer feedback forms. Here’s how it might work:
- Data Extraction: The pipeline begins by extracting raw data using SQL queries that pull information from different databases. For example:
SELECT * FROM sales WHERE sale_date >= '2024-01-01';
- Data Transformation: After extraction, transformation queries clean and structure the data. This might involve filtering out duplicates or changing data types. An example query could be:
DELETE FROM sales WHERE order_id IS NULL;
- Data Loading: Finally, the cleaned data is loaded into a destination database, ready for analysis. This might look like:
INSERT INTO cleaned_sales (product_id, sale_date, amount) SELECT product_id, sale_date, amount FROM sales;
By using a series of well-structured SQL queries, the data engineering team ensures that the pipeline runs efficiently and reliably. For a deeper look into tools for these processes, check out Building Data Pipelines: A Step-by-Step Guide 2024.
Case Study: Data Migration
Data migration is another area where SQL shines. When organizations switch from one database to another or upgrade their systems, SQL queries are pivotal in ensuring smooth transitions.
For example, let’s say a company is migrating its customer database from Oracle to PostgreSQL. During this process, SQL is crucial for managing data integrity and ensuring no information is lost. Here’s a simplified process they might follow:
- Planning the Migration: Before beginning, the team prepares by analyzing the existing database schema and ensuring compatibility with PostgreSQL.
- Extracting Data: Initial data extraction may involve SQL queries that look like this:
SELECT * FROM customers;
- Transforming Data: Next, they transform the data to fit the new schema. This might involve changing data types or restructuring tables. For instance:
INSERT INTO new_customers (id, name, email) SELECT customer_id, customer_name, customer_email FROM old_customers;
- Loading Data: Once the data is transformed, it is loaded into the new PostgreSQL database. That’s where loading queries come into play, ensuring a seamless migration.
This careful approach to SQL usage during migration mitigates risks and reduces downtime. Interested in the migration process? Check out Migration Strategies with AWS Database Migration Service for more insights.
By examining these practical applications, it’s clear how essential SQL queries are for data engineers. For additional training opportunities and hands-on practice, consider personalized training at Data Engineer Academy. For visual learners, the Data Engineer Academy YouTube channel offers valuable tutorials and examples.
Continuing Education and Resources
In the fast-paced world of data engineering, keeping your skills updated is crucial. There are numerous resources out there that can help you grow, whether through structured courses or self-paced tutorials. Here are some excellent options to consider for furthering your SQL knowledge.
Data Engineer Academy Courses
Data Engineer Academy offers personalized training opportunities that cater to your specific learning needs. This approach allows you to grasp SQL queries and other essential skills at your own pace, with the guidance of experienced professionals. If you want to take your SQL abilities to the next level, check out the personalized training programs available. These courses are designed to fit your unique learning style, making it easier to master complex concepts and improve your confidence with SQL.
YouTube Resources for SQL Learning
If you prefer visual learning, the Data Engineer Academy YouTube channel is an invaluable resource. It offers a variety of tutorials that make learning engaging and accessible. You’ll find video lessons that break down SQL concepts clearly, helping you understand how to write and execute queries effectively. Whether you’re just starting out or looking to refine your skills, these video resources can provide practical tips and real-world examples that enhance your learning experience.
Make sure to explore these avenues to expand your knowledge and enhance your career as a data engineer. By taking advantage of both structured courses and free online resources, you can build a solid foundation in SQL that will serve you well in your career.
Conclusion
Mastering SQL queries is essential for anyone pursuing a career in data engineering. The right SQL skills can streamline your daily tasks and open up opportunities for deeper analytical insights.
For those ready to enhance their SQL abilities, personalized training at Data Engineer Academy offers tailored guidance to help you grasp complex concepts effectively. Explore various topics, from fundamental queries to advanced techniques, ensuring you’re equipped for success.
Have you considered how proficient SQL skills can transform your work? The journey to becoming a top-notch data engineer starts here. Don’t forget to check out the Data Engineer Academy YouTube channel for valuable tutorials that will further boost your learning journey.
Real stories of student success
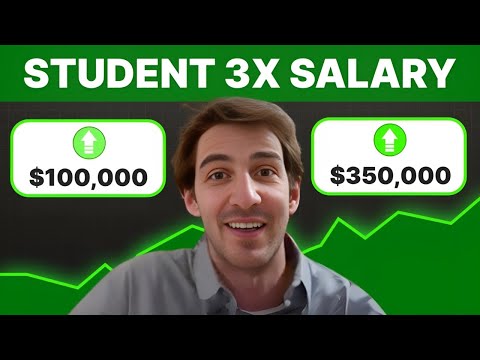
Student TRIPLES Salary with Data Engineer Academy
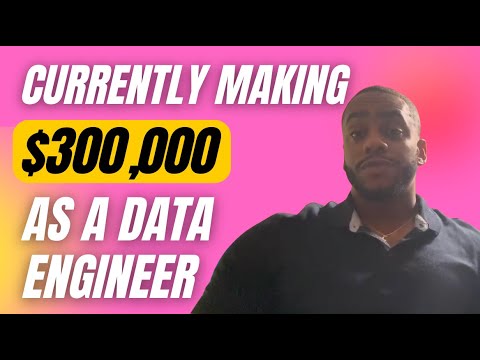
DEA Testimonial – A Client’s Success Story at Data Engineer Academy
Frequently asked questions
Haven’t found what you’re looking for? Contact us at [email protected] — we’re here to help.
What is the Data Engineering Academy?
Data Engineering Academy is created by FAANG data engineers with decades of experience in hiring, managing, and training data engineers at FAANG companies. We know that it can be overwhelming to follow advice from reddit, google, or online certificates, so we’ve condensed everything that you need to learn data engineering while ALSO studying for the DE interview.
What is the curriculum like?
We understand technology is always changing, so learning the fundamentals is the way to go. You will have many interview questions in SQL, Python Algo and Python Dataframes (Pandas). From there, you will also have real life Data modeling and System Design questions. Finally, you will have real world AWS projects where you will get exposure to 30+ tools that are relevant to today’s industry. See here for further details on curriculum
How is DE Academy different from other courses?
DE Academy is not a traditional course, but rather emphasizes practical, hands-on learning experiences. The curriculum of DE Academy is developed in collaboration with industry experts and professionals. We know how to start your data engineering journey while ALSO studying for the job interview. We know it’s best to learn from real world projects that take weeks to complete instead of spending years with masters, certificates, etc.
Do you offer any 1-1 help?
Yes, we provide personal guidance, resume review, negotiation help and much more to go along with your data engineering training to get you to your next goal. If interested, reach out to [email protected]
Does Data Engineering Academy offer certification upon completion?
Yes! But only for our private clients and not for the digital package as our certificate holds value when companies see it on your resume.
What is the best way to learn data engineering?
The best way is to learn from the best data engineering courses while also studying for the data engineer interview.
Is it hard to become a data engineer?
Any transition in life has its challenges, but taking a data engineer online course is easier with the proper guidance from our FAANG coaches.
What are the job prospects for data engineers?
The data engineer job role is growing rapidly, as can be seen by google trends, with an entry level data engineer earning well over the 6-figure mark.
What are some common data engineer interview questions?
SQL and data modeling are the most common, but learning how to ace the SQL portion of the data engineer interview is just as important as learning SQL itself.