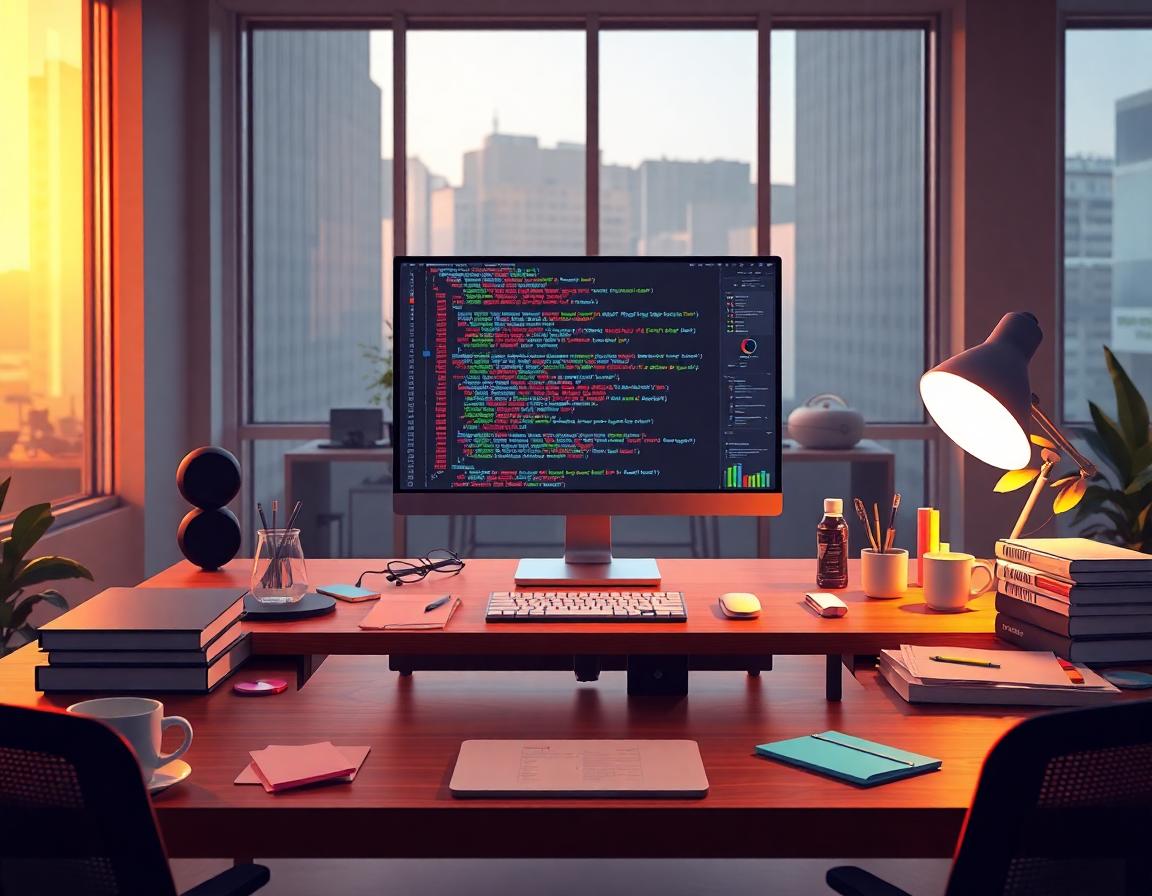
Sorting, Filtering, and Aggregating in PySpark: 10 Practice Problems to Try
Handling data efficiently is key in today’s world of big data. You might wonder: why should you care about sorting, filtering, and aggregating data in PySpark? These operations are essential for refining large datasets into meaningful insights. Whether you’re cleaning up messy information or analyzing trends, mastering these techniques can elevate your data processing skills significantly.
In this post, we’ll introduce 10 practice problems that let you apply these concepts firsthand. From simple sorting tasks to more complex aggregations, you’ll gain practical experience that you can use in real projects. Ready to boost your PySpark know-how? Let’s jump right in and tackle these practice problems together! If you’re looking for some foundational concepts before we dive deeper, check out our PySpark tutorial for beginners to get started.
Understanding Sorting in PySpark
Sorting in PySpark is an essential operation for organizing data, helping you make sense of large datasets quickly and effectively. Whether you’re preparing data for analysis or simply arranging it for better readability, mastering sorting techniques will elevate your data manipulation skills. Let’s break down how to do it step by step.
How to Sort DataFrames
Sorting DataFrames in PySpark is relatively straightforward. You can use either the sort()
function or orderBy()
method. Here’s a simple guide to help you sort your DataFrames:
- Create a DataFrame: First, ensure that you have a DataFrame to work with. You can create one from existing RDDs, CSV files, or other data sources.
- Use sort() or orderBy(): Both functions serve the same purpose. Here’s the syntax:
- Using
sort()
:df.sort("column_name")
- Using
orderBy()
:df.orderBy("column_name")
- Using
- Sort by Multiple Columns: If you want to sort by more than one column, simply pass additional column names as arguments:
df.sort("column1", "column2")
- Show Results: Finally, display your sorted DataFrame using
show()
.df.show()
For an in-depth explanation, you might want to check this detailed article on PySpark orderBy() and sort().
Sorting in Ascending and Descending Order
Sorting data in ascending and descending order is crucial for effective analysis. By default, both sort()
and orderBy()
sort in ascending order. However, you can change this by specifying the sorting order explicitly. Here’s how:
- Ascending Order: This is the default behavior, meaning you can simply use:
df.sort("column_name")
- Descending Order: To sort in descending order, use the
desc()
function:from pyspark.sql.functions import desc df.sort(desc("column_name"))
You can also mix both orders when sorting multiple columns like this:
For a real-world example, let’s say you have sales data and want to view products by highest sales first. Using descending order will provide you with quick insights.
Sorting Multiple Columns
Sorting by multiple columns allows you to organize your DataFrame based on more than one attribute. This can help uncover deeper insights. Here’s how you can achieve that in PySpark:
- Identify Columns: Determine which columns you want to use for sorting. For instance, you might want to sort first by
category
, then bysales
. - Apply Sort: Execute the sort operation:
df.sort("category", "sales")
- Specify Order: You can also control the order for each column. For example, sort by
category
in ascending order and bysales
in descending order:df.sort("category", desc("sales"))
Sorting by multiple columns can reveal patterns. For instance, you might discover that specific categories outperform others in sales, leading to better strategic decisions.
Sorting, filtering, and aggregating data in PySpark plays a crucial role in data processing and analysis. To enhance your skills, consider practicing these techniques using the Ultimate PySpark Practice Questions. With these foundational concepts under your belt, you’ll be ready to tackle more complex data operations!
Filtering Data in PySpark
Filtering data in PySpark is a pivotal operation that allows you to extract specific rows from your DataFrame based on criteria that matter most to you. In a world where data can become overwhelming, filtering helps manage your datasets by isolating the information that’s relevant to your analysis. Let’s break down how to make the most of filtering in PySpark through various approaches.
Using the Filter Function
The filter()
function in PySpark is your go-to for retrieving specific entries from a DataFrame. It allows you to apply a condition, and only those entries meeting this condition will be returned. Here’s a clear example of how to use it:
- Basic Usage: To filter entries, you simply call the
filter()
method on your DataFrame.filtered_df = df.filter(df.column_name == "value")
This will return all rows wherecolumn_name
matches the specified value. - Using SQL Expressions: PySpark also allows SQL-like syntax for filtering:
filtered_df = df.filter("column_name = 'value'")
Whether you’re identifying sales over a certain threshold or isolating entries from a specific region, the filter()
function makes it straightforward. For an in-depth view on filtering, consider checking out PySpark where() & filter() for efficient data filtering.
Conditional Filtering
Now, let’s look into conditional filtering. This approach allows you to implement various conditional statements, giving you a powerful way to refine your dataset. For instance:
- Single Condition: You might want specific rows based on a single criterion, like this:
filtered_df = df.filter(df.age > 30)
- Multiple Conditions: Using logical operators, you can easily apply multiple conditions:
filtered_df = df.filter((df.age > 30) & (df.salary < 50000))
Here, we’re pulling entries where age is over 30 and salary is below 50,000. Easy, right?
Conditional filtering is majorly useful in scenarios such as filtering customer data, where you might want to target users based on age and income levels, making your analysis not only targeted but hyper-relevant.
Combining Filters with Logical Operators
Combining multiple filter conditions can enhance your data extraction process significantly. In PySpark, you can utilize logical operators like AND and OR to create more complex filtering scenarios.
- Using AND: This operator requires all conditions to be true. For example:
filtered_df = df.filter((df.age < 25) & (df.city == 'New York'))
In this case, you’re retrieving data for individuals younger than 25 who live in New York. - Using OR: With this operator, only one condition needs to be satisfied:
filtered_df = df.filter((df.age < 25) | (df.city == 'Los Angeles'))
Here, you’re pulling in data for young people or those living in Los Angeles.
Using these logical combinations can significantly elevate how you analyze data by allowing for nuanced queries. Want to learn how to filter with multiple conditions? Check out this insightful article on how to filter a DataFrame based on multiple conditions.
By mastering filtering in PySpark, you’re equipped to hone in on the data that truly matters to your analysis. Take your time to experiment with these functions, and you’ll quickly find them invaluable in your data processing toolkit!
. Photo by Lukas
Aggregating Data in PySpark
When it comes to analyzing datasets in PySpark, aggregation is at the heart of turning raw numbers into meaningful insights. Whether you’re computing totals, averages, or counts, knowing how to aggregate data efficiently is essential for any data-driven project. This section will walk you through basic aggregation functions, how to group your data for effective aggregation, and how to perform complex queries that combine multiple aggregations.
Aggregation Functions: SUM, AVG, COUNT
In PySpark, understanding the fundamental aggregation functions like SUM
, AVG
, and COUNT
is vital. These functions help summarize data across multiple rows effectively.
- SUM: This function adds up all of the values in a given column, allowing you to quickly determine totals. For example, if you’re running a sales analysis, you might want to find the total sales amount:
total_sales = df.agg({"sale_amount": "sum"})
- AVG: The average function calculates the mean of the values in a specified column. This is useful for understanding metrics like average sale price or average customer age:
average_age = df.agg({"age": "avg"})
- COUNT: Count returns the number of rows in a dataset or the number of non-null entries for a specific column. This function is particularly useful for understanding the volume of data you are dealing with:
customer_count = df.agg({"customer_id": "count"})
These aggregation functions are powerful tools for data summarization. To learn more about detailed aggregation use cases, you can check out the PySpark Aggregate Functions with Examples.
Grouping Data for Aggregation
The groupBy
method in PySpark is pivotal for aggregation, allowing you to manage datasets effectively by grouping them according to specific columns. Here’s how you can do this:
- Define Groups: Use the
groupBy
method to specify the columns you want to group by. For instance:grouped_data = df.groupBy("category")
- Apply Aggregations: Once you’ve defined your groups, you can apply your aggregation functions directly. Here’s an example of calculating total sales per category:
category_sales = grouped_data.agg({"sale_amount": "sum"})
- Show Results: Display the aggregate results to visualize your data:
category_sales.show()
Grouping data is especially effective when you’re analyzing trends across different segments. Want hands-on examples? Consider reviewing the article on PySpark Data Aggregation: A Comprehensive Guide.
Using Aggregations with Complex Queries
How can you combine multiple aggregations in a single query? It’s easier than you think! Complex queries can provide deeper insights into your data. Let’s explore an example:
Imagine you want to analyze sales performance across different categories by calculating both the total sales and average price for each category. Here’s how you could do it:
- Group and Compute: First, group by the relevant column and then apply multiple aggregations:
aggregated_data = df.groupBy("category").agg( {"sale_amount": "sum", "sale_price": "avg"} )
- Filter Results: You can further refine your results by filtering. For instance, if you’re interested only in categories with total sales above a certain threshold:
filtered_results = aggregated_data.filter(aggregated_data["sum(sale_amount)"] > 1000)
- Display Final Output: Finally, show the refined results:
filtered_results.show()
Combining these aggregation functions can unlock valuable insights, enabling you to answer questions like, “Which product categories are performing best?” or “What are the average sales prices across segments?”
In data analysis, the ability to perform aggregations accurately can significantly impact your decision-making process. For a deeper dive into complex queries and examples, don’t miss the Medium article on PySpark Aggregate Functions.
Photo by Pixabay
10 Practice Problems to Enhance Your Skills
To help you get hands-on experience sorting, filtering, and aggregating in PySpark, here are ten practice problems that you can work through. These problems range from basic to advanced levels, allowing you to build confidence as you tackle each task. Let’s dive into the details!
Problem 1: Simple Sorting Task
Your task is to create a DataFrame containing the following employee details: name
, age
, and salary
. Now, sort the DataFrame based on the salary
column in ascending order. This will help you quickly identify your lowest-paying employees.
Problem 2: Multiple Column Sorting
Imagine you have a DataFrame with columns product
, category
, and price
. Create a scenario where you need to sort first by category
in ascending order and then by price
in descending order. How does this arrangement inform your pricing strategy for different product categories?
Problem 3: Basic Filtering Task
Design a problem where you filter a dataset of student grades that contains student_id
, name
, score
. Your objective is to return all records where the score is greater than or equal to 70. This will help identify students who are passing.
Problem 4: Conditional Filters
Create a more complex challenge that requires filtering a DataFrame of customer data containing customer_id
, age
, and purchases
. Implement a filter where you only return customers aged between 25 and 35 who have made more than 5 purchases. This is a great way to identify your active customer segment.
Problem 5: Data Aggregation Challenge
You have a sales dataset containing order_id
, product
, and amount
. Your challenge is to calculate the total sales amount for each product. Group the data by product
and find the sum of amount
. What insights can this aggregate information provide about your inventory?
These problems are designed not only to deepen your understanding of PySpark but also to encourage active exploration of the language’s capabilities. Need more practice? Check out the 100+ Python Coding Questions for Data Engineers to further sharpen your skills!
Conclusion
Sorting, filtering, and aggregating data in PySpark equips you with essential skills for effective data analysis and manipulation. By mastering these techniques through hands-on practice, you’ll gain confidence in handling large datasets and uncovering valuable insights.
For those looking to take a deeper dive into the world of PySpark, revisiting the fundamental concepts covered in the PySpark tutorial for beginners will strengthen your foundation.
Additionally, don’t miss out on the chance to further enhance your skills with the Ultimate PySpark Practice Questions.
What challenges have you faced when working with PySpark? Share your experiences, and let’s engage in a discussion about the best practices and tips you’ve discovered!
Real stories of student success
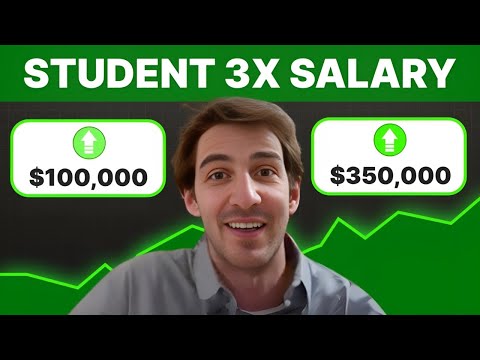
Student TRIPLES Salary with Data Engineer Academy
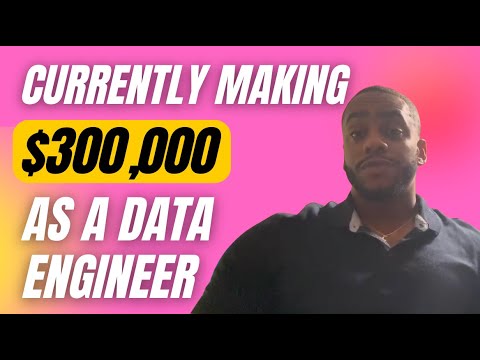
DEA Testimonial – A Client’s Success Story at Data Engineer Academy
Frequently asked questions
Haven’t found what you’re looking for? Contact us at [email protected] — we’re here to help.
What is the Data Engineering Academy?
Data Engineering Academy is created by FAANG data engineers with decades of experience in hiring, managing, and training data engineers at FAANG companies. We know that it can be overwhelming to follow advice from reddit, google, or online certificates, so we’ve condensed everything that you need to learn data engineering while ALSO studying for the DE interview.
What is the curriculum like?
We understand technology is always changing, so learning the fundamentals is the way to go. You will have many interview questions in SQL, Python Algo and Python Dataframes (Pandas). From there, you will also have real life Data modeling and System Design questions. Finally, you will have real world AWS projects where you will get exposure to 30+ tools that are relevant to today’s industry. See here for further details on curriculum
How is DE Academy different from other courses?
DE Academy is not a traditional course, but rather emphasizes practical, hands-on learning experiences. The curriculum of DE Academy is developed in collaboration with industry experts and professionals. We know how to start your data engineering journey while ALSO studying for the job interview. We know it’s best to learn from real world projects that take weeks to complete instead of spending years with masters, certificates, etc.
Do you offer any 1-1 help?
Yes, we provide personal guidance, resume review, negotiation help and much more to go along with your data engineering training to get you to your next goal. If interested, reach out to [email protected]
Does Data Engineering Academy offer certification upon completion?
Yes! But only for our private clients and not for the digital package as our certificate holds value when companies see it on your resume.
What is the best way to learn data engineering?
The best way is to learn from the best data engineering courses while also studying for the data engineer interview.
Is it hard to become a data engineer?
Any transition in life has its challenges, but taking a data engineer online course is easier with the proper guidance from our FAANG coaches.
What are the job prospects for data engineers?
The data engineer job role is growing rapidly, as can be seen by google trends, with an entry level data engineer earning well over the 6-figure mark.
What are some common data engineer interview questions?
SQL and data modeling are the most common, but learning how to ace the SQL portion of the data engineer interview is just as important as learning SQL itself.